What is the difference between a prototype and a constructor in TypeScript?
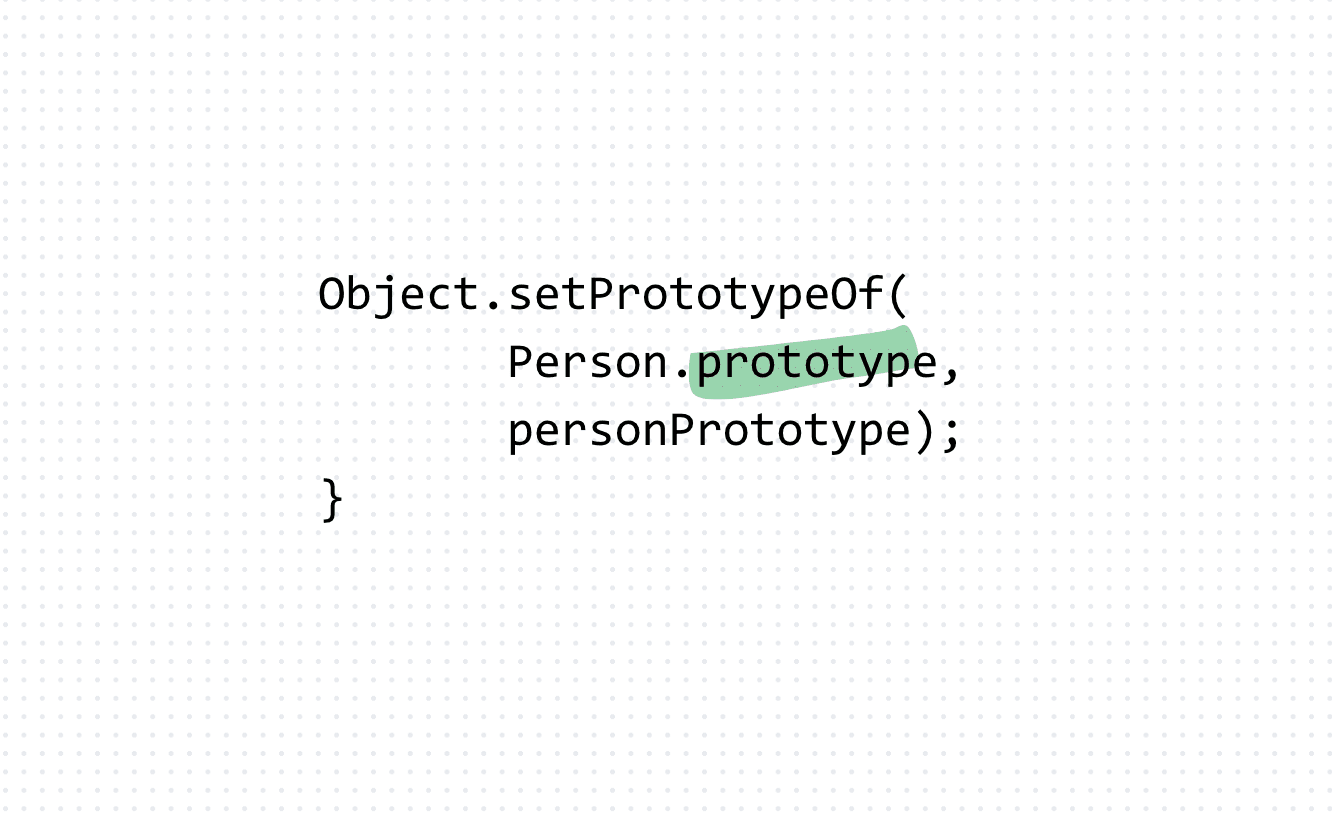
A constructor is a special method in a class that is called when an object is created from the class. It is responsible for initializing the object's properties and setting up its internal state. In JavaScript, a constructor is typically denoted by the constructor keyword.
class Person {
constructor(name) {
this.name = name;
}
}
const person = new Person('John');
console.log(person.name);
A prototype is an object that serves as a template for creating new objects. It is essentially a blueprint that defines the properties and methods of an object. In JavaScript, every object has a prototype, which is used to create new objects.
class Person {
constructor(name) {
this.name = name;
}
}
const personPrototype = {
sayHello() {
console.log(`Hello, my name is ${this.name}!`);
}
};
Object.setPrototypeOf(Person.prototype, personPrototype);
const john = new Person('John');
john.sayHello();
In this example, we define a Person class with a constructor, and then create a separate object personPrototype that defines a sayHello method. We then set the prototype of the Person class to personPrototype using Object.setPrototypeOf. This allows us to add the sayHello method to all Person objects.
In summary, constructors and prototypes are two distinct concepts in JavaScript. While constructors are used to initialize objects, prototypes are used to define the properties and methods of objects. Understanding the difference between the two is essential for building robust and maintainable JavaScript applications.