Roman to Integer Algorithm
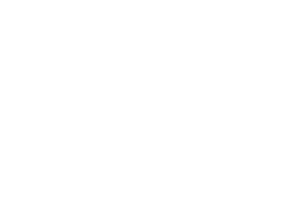
Roman numerals are a numeral system originating from ancient Rome, consisting of seven symbols: I (1), V (5), X (10), L (50), C (100), D (500), and M (1000). By combining these symbols in different ways, the Romans represented numbers. For example, III represents 3, XIV represents 14, and MMXIX represents 2019. Converting Roman numerals to integers involves deciphering the symbolic representation and calculating the corresponding numerical value.
The Roman to Integer algorithm aims to convert a given Roman numeral string to its equivalent integer value. Let's explore a simple and efficient technique using JavaScript:
Let's code:
function romanToInt(s) {
const romanValues = {
'I': 1,
'V': 5,
'X': 10,
'L': 50,
'C': 100,
'D': 500,
'M': 1000
};
let newValue = 0;
for ( let i = 0; i < s.length; i+=1) {
if (s[i+1] && romanValues[s[i+1]] > romanValues[s[i]]) {
newValue -= romanValues[s[i]]
} else {
newValue += romanValues[s[i]]
}
}
return newValue;
}
// Example usage
romanToInt("III") // 3
romanToInt("XIV") // 14
romanToInt("MMXIX") // 2019
- We create a mapping of Roman numeral characters to their corresponding integer values.
- We iterate through the input Roman numeral string and calculate the integer value based on the symbols' positions and values.
- If a smaller value precedes a larger value (e.g., IV for 4), we subtract the smaller value from the larger one to account for the special case.
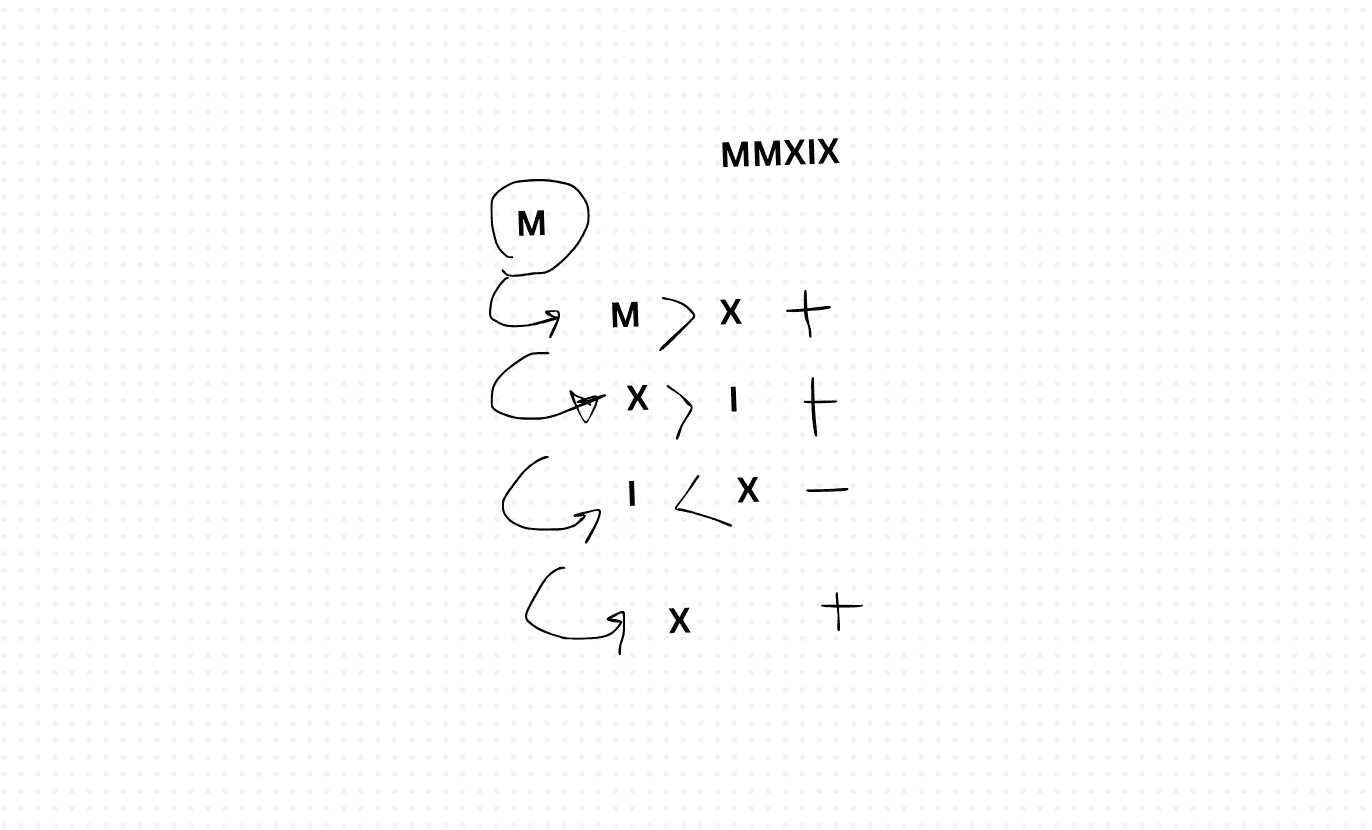
Conclusion
The Roman to Integer algorithm, with its blend of historical significance and mathematical intricacy, represents a captivating challenge in the realm of computer science.