Two Sum Algorithm
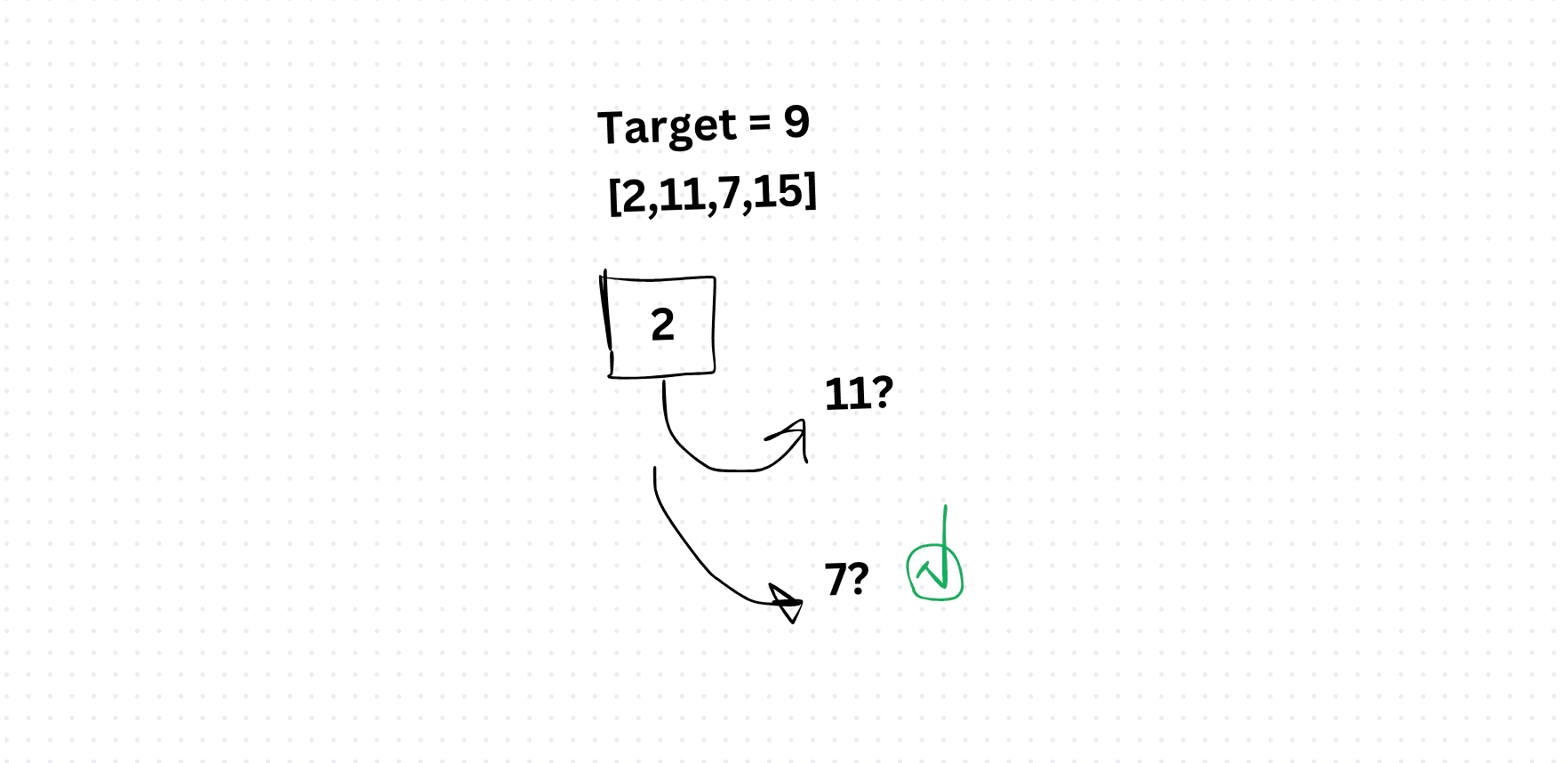
Imagine you have a list of numbers and a target sum. The task is simple: find two numbers in the list that add up to the target. For example, if you have [2, 7, 11, 15] and need to sum up to 9, the answer is [0, 1] (2 + 7 = 9).
Let's Code it Up!
Here's a JavaScript solution using nested loops:
function twoSum(nums, target) {
for (let i = 0; i < nums.length; i++) {
for (let j = i + 1; j < nums.length; j++) {
if (nums[i] + nums[j] === target) {
return [i, j];
}
}
}
return null;
}
// Example usage
const nums = [2, 7, 11, 15];
const target = 9;
console.log(twoSum(nums, target)); // Output: [0, 1]
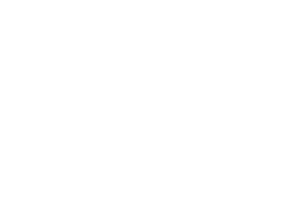
The Two Sum algorithm isn't as daunting as it seems. With a bit of hashmap magic, you're ready to conquer coding interviews and tackle real-world challenges alike. So, next time you're faced with a Two Sum puzzle, remember: hashmap to the rescue!
More Efficient:
const twoSum = function(numbers, target) {
let leftIndex = 0;
let rightIndex = numbers.length - 1;
while (leftIndex < rightIndex) {
const sum = numbers[leftIndex] + numbers[rightIndex];
if (sum === target) {
return [leftIndex + 1, rightIndex + 1];
} else if (sum < target) {
leftIndex++;
} else {
rightIndex--;
}
}
return [-1, -1]; // No such pair found
};