Contrast between React Lazy Load and React Code Splitting
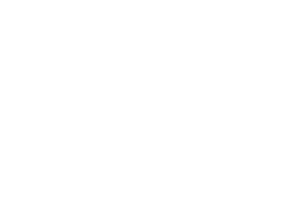
React lazy loading is a technique used to defer the loading of components until they are needed, typically during navigation or user interaction. This helps reduce the initial bundle size and improves the loading time of the application. Lazy loading is particularly useful for large applications with complex routing and multiple views.
To implement lazy loading in a TypeScript React application, use the React.lazy() function to dynamically import components. Here's an example:
import React, { Suspense } from 'react';
const LazyComponent = React.lazy(() => import('./LazyComponent'));
const MyLazyComponent: React.FC = () => (
<Suspense fallback={<div>Loading...</div>}>
<LazyComponent />
</Suspense>
);
To implement code splitting in a TypeScript React application, use dynamic imports to split your code into smaller chunks. Here's an example:
import React, { useState } from 'react';
const MyComponent: React.FC = () => {
const [isModuleLoaded, setIsModuleLoaded] = useState(false);
const loadModule = async () => {
const module = await import('./DynamicModule');
setIsModuleLoaded(true);
};
return (
<div>
<button onClick={loadModule}>Load Module</button>
{isModuleLoaded && <DynamicModule />}
</div>
);
};
Difference between React Lazy Loading and Code Splitting
The primary difference between React lazy loading and code splitting lies in their purposes and implementation:
- React Lazy Loading: Lazy loading defers the loading of components until they are needed, improving performance by reducing the initial bundle size. It is typically used for individual components or routes.
- React Code Splitting: Code splitting breaks down the application's bundle into smaller chunks, loading them asynchronously as needed. It optimizes performance by only loading the necessary code for the current view or route, making it suitable for larger applications with complex routing.
In TypeScript React applications, both lazy loading and code splitting are essential techniques for optimizing performance and enhancing user experience. While lazy loading defers the loading of components until they are needed, code splitting breaks down the application's bundle into smaller chunks, loading them asynchronously. By understanding and leveraging these techniques effectively, developers can create faster, more responsive React applications, providing a seamless user experience.