Digit Recogniser Machine Learning Tensorflow Javascript And React
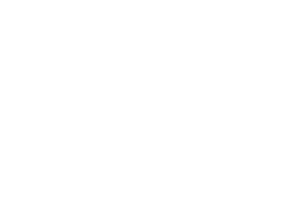
I wanted to develop Digit Recognition using Machine Learning, and this post is about my experience in learning and implementing Digit Recognition using Tensorflow JavaScript I also created a GitHub repository.
Github: https://github.com/ehsangazar/digit-recogniser-machine-learning-tensorflow-javascript
Demo: https://ehsangazar.github.io/digit-recogniser-machine-learning-tensorflow-javascript/
Neural networks in machine learning or ANN ( Artificial Neural Network ) is the way of defining a network and starting training data to help machines learn new patterns.
Artificial neural networks (ANNs) or connectionist systems are computing systems vaguely inspired by the biological neural networks that constitute animal brains
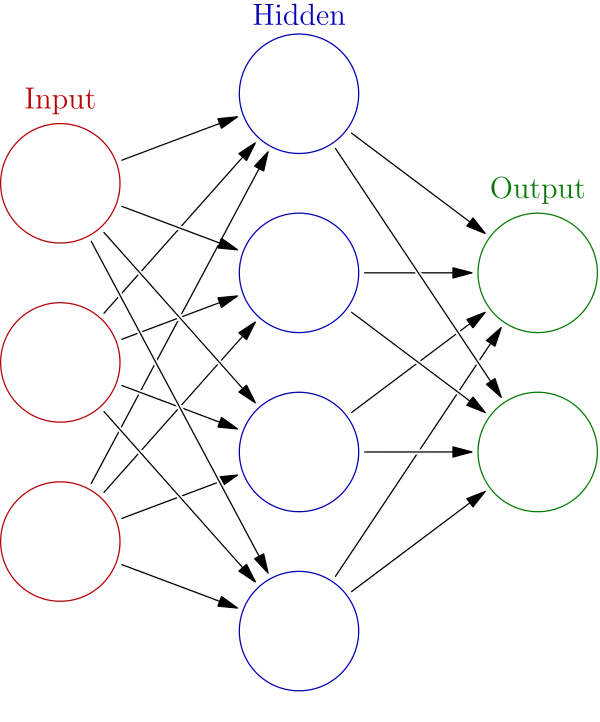
We simply try to create these hidden layers for understanding Inputs to match it to possible outputs.
An artificial neural network is an interconnected group of nodes, akin to the vast network of neurons in a brain. Here, each circular node represents an artificial neuron and an arrow represents a connection from the output of one artificial neuron to the input of another.
You can use Playground of Tensorflow for having more visual understanding of layers and inputs.
One of the popular Artificial Neural Networks is CNN or Convolutional neural network. The special thing about these type of network is their Convolutional layouts
In machine learning, a convolutional neural network (CNN, or ConvNet) is a class of deep, feed-forward artificial neural networks, most commonly applied to analyzing visual imagery. CNNs use a variation of multilayer perceptrons designed to require minimal preprocessing.[1] They are also known as shift invariantor space invariant artificial neural networks (SIANN), based on their shared-weights architecture and translation invariancecharacteristics.[2][3]
Convolutional Neural Networks or CNN are for solving classification problems, which means recognising a pattern and match it to possible results.
In this kind of network, each layer convolute the initial data and at the end it can convert to the result.
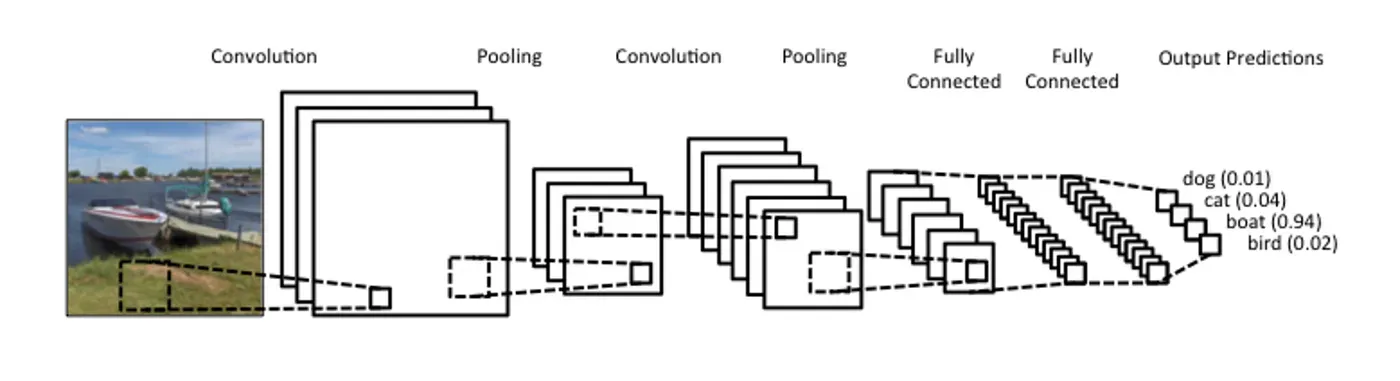
So, after understanding this, it is kind of obvious that Digit Recognition can be solved with the same network.
Everything starts with installing Tensorflow:
npm install @tensorflow/tfjs
then I imported it:
import * as tf from '@tensorflow/tfjs';
I created a sequential model:
// Creates a tf.Sequential model.A sequential model is any model where the outputs of one
// layer are the inputs to the next layer,
this.minstModel = tf.sequential();
Then I needed to define the layers, which Tensorflow has Convolutional layers, with conv2d function. Conv2d function helps us to understand images which they are two dimensional.
this.minstModel.add(tf.layers.conv2d({
inputShape: [28, 28, 1],
kernelSize: 5,
filters: 8,
strides: 1,
activation: 'relu',
kernelInitializer: 'varianceScaling'
}));
this.minstModel.add(tf.layers.maxPooling2d({
poolSize: [2, 2],
strides: [2, 2]
}));
this.minstModel.add(tf.layers.conv2d({
kernelSize: 5,
filters: 16,
strides: 1,
activation: 'relu',
kernelInitializer: 'varianceScaling'
}));
this.minstModel.add(tf.layers.maxPooling2d({
poolSize: [2, 2],
strides: [2, 2]
}));
this.minstModel.add(tf.layers.flatten());
this.minstModel.add(tf.layers.dense({
units: 10,
kernelInitializer: 'varianceScaling',
activation: 'softmax'
}));
As you see in the code above, there are different layers like maxPooling2d , flatten and dense
MaxPooling2d layer is for performing down-sampling by dividing the input into rectangular pooling regions, and computing the maximum of each region.
Flatten layer is for making use of fully connected layers after some convolutional layers
Dense layer represents a matrix vector multiplication.
So you can understand from the model, we are trying to map our images with a Conv2d layer and then sampling it with a MaxPooling2d. Therefor we use Conv2d and MaxPooling for making our inputs even smaller and using flatten and dense at the end will help us to get the loss using Softmax.
You might think about this question that how can you develop this layers, it needs a deep level of understanding each one of these and it is so hard to make them efficient. That is a reason you can compete with others in Kaggle here.
If you open the demo:
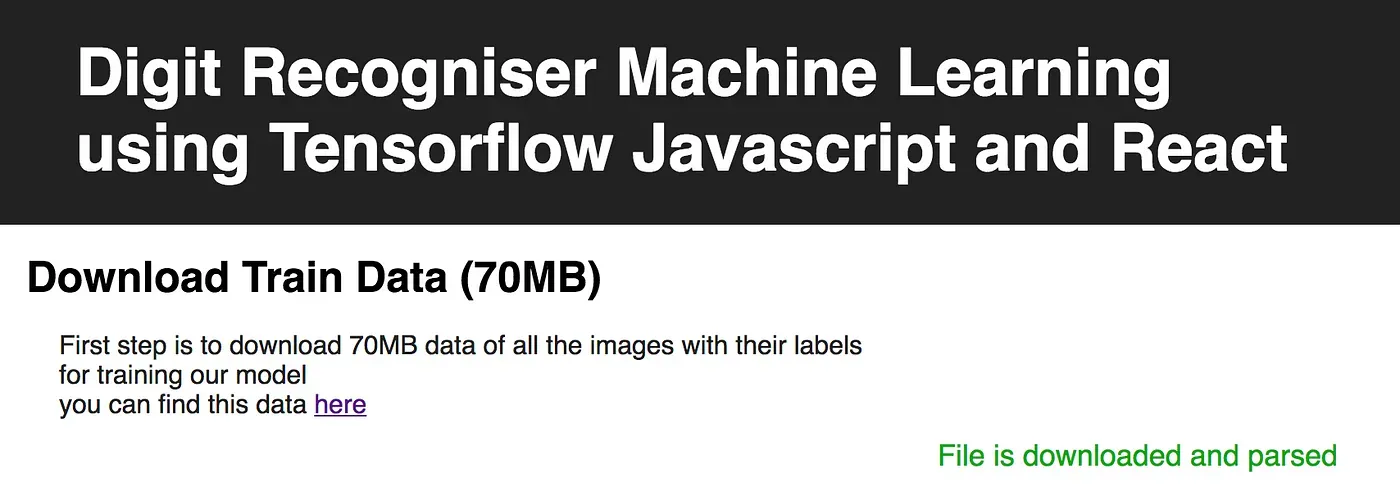
__ Read the training data using _readTrainingData
First we need to download all the data from train.csv to our project and use papaparse for parsing the CSV to array. This data includes number of pixels with their information.
__ Initialising the model using _initializingTheModel
After downloading the information, we need to define our model which is a sequential model and we want to use SGD, but for using SGD, we need a loss function and layers of model plus an optimizer.
SGD can use our model definition to train the data. SGD is one of the most common training methods in Machine Learning which is being used for Large datasets
__ Start Training _trainNewModel

After initializing the model, you can use this function for start the training, because the training data is so big, we have to use part of the file each time to train the model. It takes a while to train the model and in a meantime you can see the log in your console.
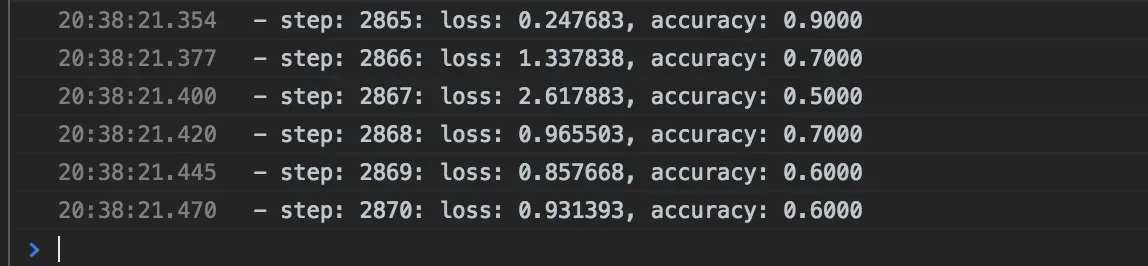
const history = await this.minstModel.fit(
batch.inputs,
batch.labels,
{
batchSize: batchSize
}
);
__ Evaluating our model using _minscPredictionLabeled
And finally for evaluating our model, I didn’t use the 100 inputs of training data and I developed to use it for knowing the accuracy or our model.
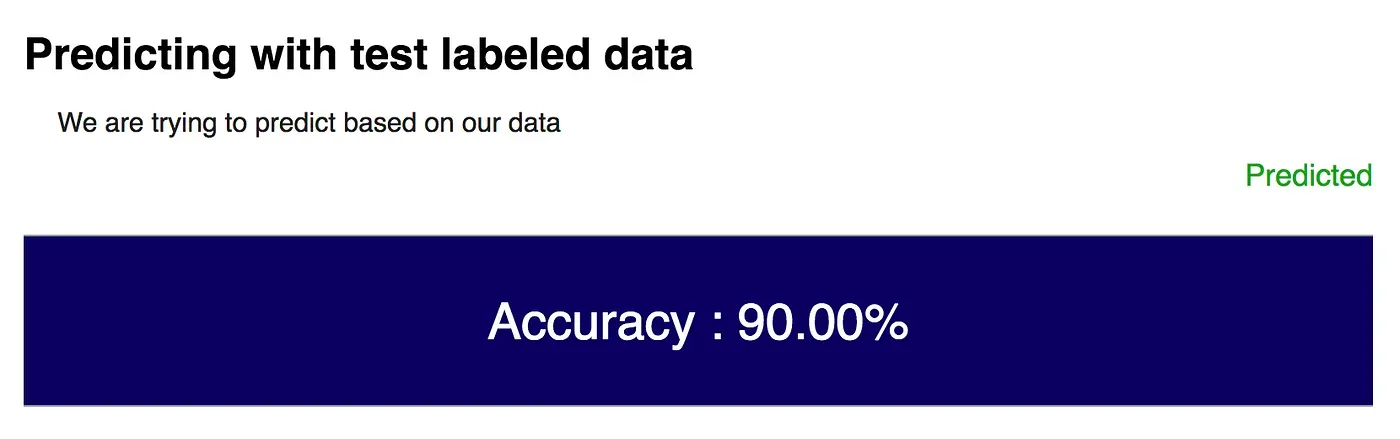
__ Canvas Prediction
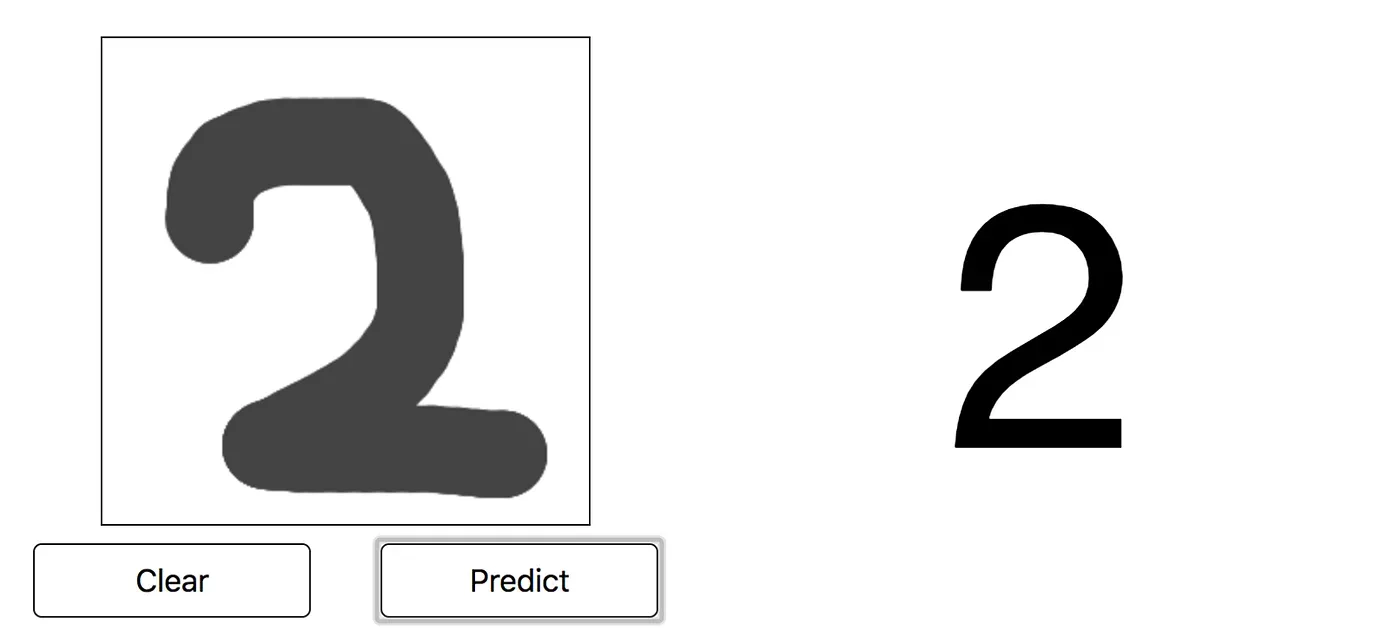
For visualizing this training, I added a Canvas to the project which eventually creates an image of 28 * 28 pixels and uses the model to predict the number
__ Kaggle Competition
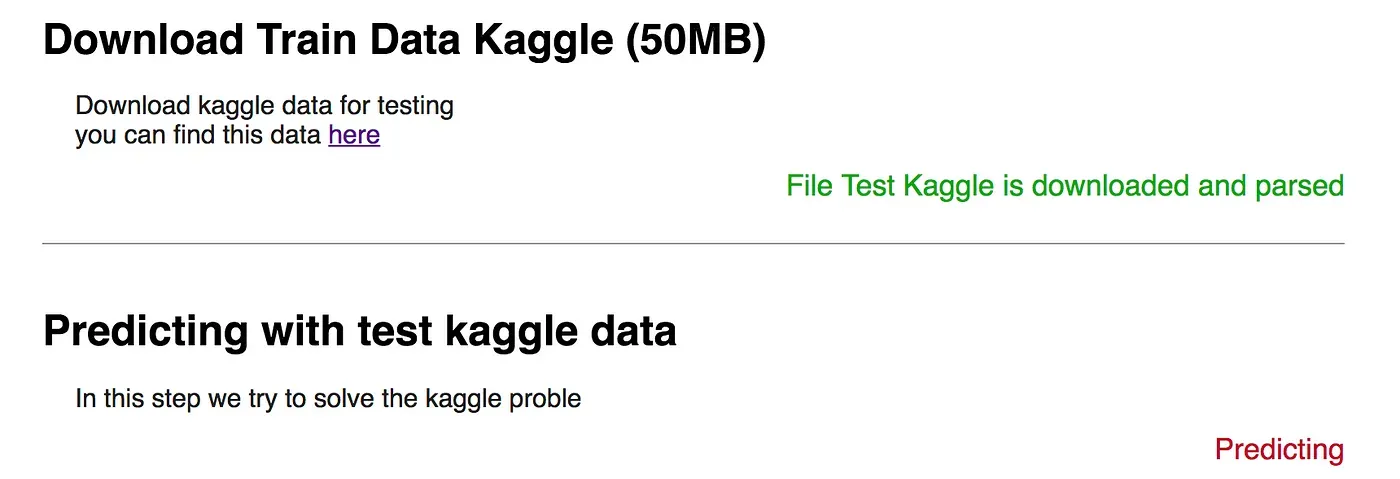
End of this demo is for trying to solve the Kaggle test data. The output can be used to submit as a solution for the competition.
Final Note:
The more you read about Machine Learning, you will understand no matter how many years scientists have been working on it, there are still so many things to discover.
This projects shows for being an expert in machine learning you need to spend few years to learn those algorithms and libraries to be able to design a useful model.