Top GraphQL Interview Questions with Code Examples
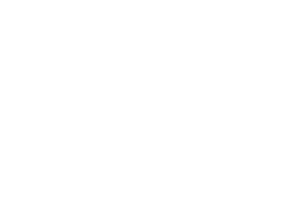
GraphQL has become a popular choice for building APIs due to its flexibility and efficiency in data retrieval. In interviews for GraphQL-related roles, candidates are often tested on their understanding of key concepts and their ability to implement solutions using GraphQL. In this article, we'll explore 20 common GraphQL interview questions along with code examples to help you prepare effectively.
What is GraphQL and how does it differ from REST?
GraphQL is a query language for APIs that enables clients to request exactly the data they need.
Unlike REST APIs, which expose predefined endpoints, GraphQL allows clients to specify the shape and structure of the data they require in a single request.
Explain GraphQL Schema and its types.
GraphQL Schema defines types and relationships in the API. Types include objects, scalars, enums, and interfaces.
type User {
id: ID!
name: String!
email: String!
}
type Query {
user(id: ID!): User
}
What are the benefits of using GraphQL over REST?
Scalar types in GraphQL represent primitive data types like String, Int, Float, Boolean, and ID. They are the building blocks for defining the shape of data in GraphQL schemas.
- String: A sequence of characters.
- Int: A signed 32-bit integer.
- Float: A signed double-precision floating-point value.
- Boolean: Represents true or false.
- ID: A unique identifier, often serialized as a string.
GraphQL also allows defining custom scalar types, which provide a way to handle and enforce specific data formats or semantics beyond the built-in scalar types.
scalar Date type Event {
id: ID!
title: String!
date: Date!
}
What is a resolver function in GraphQL?
Resolver functions are responsible for fetching the data for a particular field in a GraphQL query.
const resolvers = {
Query: {
user: (parent, { id }, context) => {
// Logic to fetch user data from the database
}
}
};
How to perform mutations in GraphQL?
Mutations are used to modify data on the server. They are similar to queries but are used for create, update, or delete operations.
mutation {
createUser(name: "John", email: "john@example.com") {
id
name
email
}
}
What is a fragment in GraphQL?
GraphQL fragments allow developers to define reusable sets of fields that can be included in queries.
fragment UserInfo on User {
id
name
email
}
What are union types in GraphQL? Provide an example.
Union types allow a field to return more than one type of object.
union SearchResult = User | Product | Post
How to implement authentication and authorization in GraphQL?
Authentication and authorization in GraphQL can be implemented using middleware or by adding context to resolver functions to check user permissions.
Explain resolver chaining in GraphQL.
Resolver chaining is the process of resolving nested fields in a GraphQL query by chaining resolver functions.
const resolvers = {
Query: {
user: (parent, { id }, context) => {
// Logic to fetch user data from the database
}
},
User: {
posts: (parent, args, context) => {
// Logic to fetch posts associated with the user
}
}
};
How do you handle file uploads in GraphQL?
Handling file uploads in GraphQL typically involves a multi-step process, as GraphQL itself does not natively support file uploads. Here's a general approach to handle file uploads in a GraphQL server:
type Mutation {
uploadFile(file: Upload!): File!
}
Then you can have a resolver like this:
const resolvers = {
Mutation: {
uploadFile: async (_, { file }) => {
// Ensure directory for file uploads exists
mkdirSync('./uploads', { recursive: true });
// Create a writable stream to save the file
const stream = createWriteStream(`./uploads/${file.filename}`);
// Pipe the file data from the client to the writable stream
await new Promise((resolve, reject) => {
file.createReadStream()
.pipe(stream)
.on('finish', resolve)
.on('error', reject);
});
// Return metadata about the uploaded file
return {
filename: file.filename,
mimetype: file.mimetype,
encoding: file.encoding,
url: `http://example.com/uploads/${file.filename}`
};
}
}
};
What is introspection in GraphQL, and how is it useful?
Introspection in GraphQL refers to the ability of a GraphQL server to provide information about its schema and types at runtime. It allows clients to query the server's schema and discover the available fields, types, arguments, and directives. This feature is built into GraphQL and is used by various tools and libraries.
What are directives in GraphQL, and how do you use them?
Some of the most commonly used built-in directives include @include, @skip, and @deprecated.
- @include(if: Boolean): Conditionally includes a field in the result based on the value of the provided boolean argument.
- @skip(if: Boolean): Conditionally skips a field in the result based on the value of the provided boolean argument.
- @deprecated(reason: String): Marks a field as deprecated and provides a deprecation reason.
query {
user(id: "123") {
id
name
email @include(if: true)
age @skip(if: false)
}
}
What is the difference between GraphQL subscriptions and WebSocket communication?
GraphQL subscriptions and WebSocket communication are related concepts but serve different purposes. GraphQL subscriptions allow clients to receive real-time updates from the server when certain events occur, providing a mechanism for real-time data push. Subscriptions are typically used for scenarios like real-time collaboration, notifications, or live feeds.
On the other hand, WebSocket is a communication protocol that establishes a persistent connection between the client and the server. It enables full-duplex, bidirectional communication, making it ideal for implementing GraphQL subscriptions. While GraphQL subscriptions utilize WebSocket as the transport, GraphQL itself is still based on HTTP, and queries and mutations are sent via standard HTTP POST requests.
Conclusion
Preparing for GraphQL interviews can be challenging, but mastering these common questions and understanding the underlying concepts will set you on the path to success. By practicing with these code examples and delving deeper into GraphQL documentation and tutorials, you'll be well-equipped to tackle any GraphQL interview with confidence. Happy coding!