React Error Boundary in a TypeScript React Application
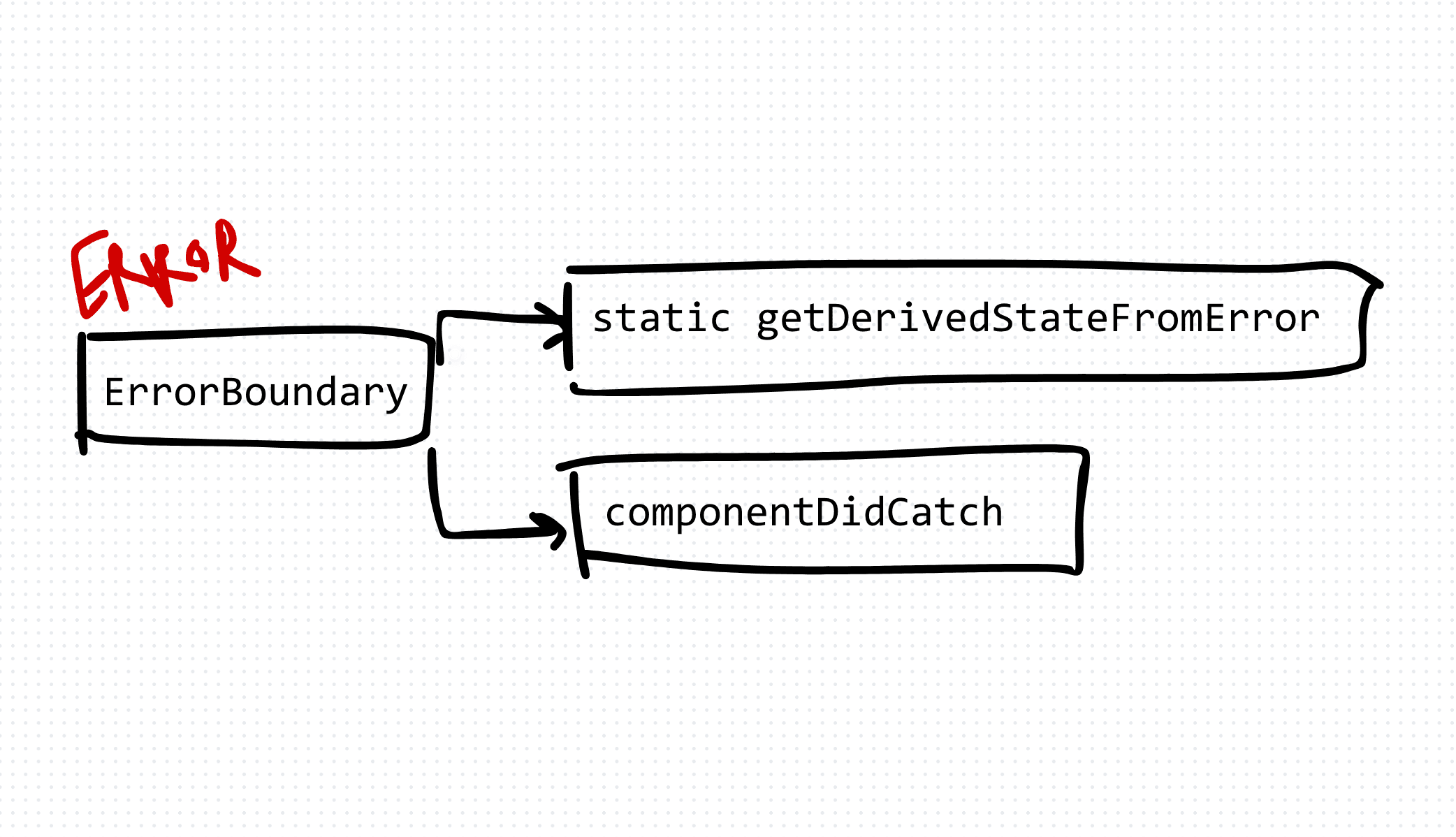
React error boundaries are special components that catch errors that occur during rendering, in lifecycle methods, and in constructors of the whole component tree below them. They are used to encapsulate components that may throw errors and prevent those errors from propagating up the component tree, thus preventing the entire application from crashing.
To implement a React error boundary in a TypeScript React application, follow these steps:
- Create an Error Boundary Component: Define a class component that extends React.Component and implements the componentDidCatch lifecycle method to catch errors.
- Use the Error Boundary Component: Wrap the components that you want to be covered by the error boundary with the error boundary component.
interface ErrorBoundaryPropsTypes {
children: React.ReactNode;
fallback: React.ReactNode;
}
interface ErrorBoundaryStateTypes {
hasError: boolean
}
class ErrorBoundary extends React.Component<
ErrorBoundaryTypes,
ErrorBoundaryStateTypes
> {
constructor(props: ErrorBoundaryTypes) {
super(props);
this.state = { hasError: false };
}
static getDerivedStateFromError(error: Error) {
return { hasError: true };
}
componentDidCatch(error: Error, errorInfo: React.ErrorInfo) {
console.error(error, errorInfo);
}
render() {
if (this.state.hasError) {
return this.props.fallback;
}
return this.props.children;
}
}
In TypeScript React applications, implementing error boundaries provides a robust mechanism for handling errors and preventing the entire application from crashing due to unexpected errors. By encapsulating error-prone components within error boundaries, developers can ensure a smoother user experience and easier debugging. Understanding and utilizing error boundaries effectively enables developers to build more resilient and reliable React applications.