Power of gRPC in Node.js: A Superior Alternative to Websockets
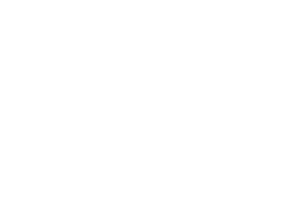
gRPC utilizes HTTP/2, a binary protocol, which offers significant performance benefits over the text-based protocols like HTTP/1.1 used by Websockets. This results in lower latency and higher throughput, making gRPC ideal for demanding real-time applications.
gRPC relies on Protocol Buffers (protobuf) for defining service contracts, which provides strong typing and automatic code generation in multiple languages. This ensures better communication between client and server, reducing the likelihood of runtime errors compared to the more flexible but error-prone nature of Websockets.
gRPC supports bidirectional streaming, allowing both the client and server to send a stream of messages asynchronously. This feature is particularly useful for scenarios like chat applications, telemetry systems, and real-time analytics, where constant data exchange is required.
1. Define the Protocol Buffer (.proto) File:
syntax = "proto3";
service MyService {
rpc SendMessage(Message) returns (Response);
}
message Message {
string content = 1;
}
message Response {
string reply = 1;
}
Use the protoc compiler with the Node.js plugin to generate client and server code from the .proto file.
NodeJS
const grpc = require('grpc');
const protoLoader = require('@grpc/proto-loader');
const { sendMessage } = require('./controller');
const PROTO_PATH = __dirname + '/path/to/proto/file.proto';
const packageDefinition = protoLoader.loadSync(PROTO_PATH);
const { MyService } = grpc.loadPackageDefinition(packageDefinition);
function main() {
const server = new grpc.Server();
server.addService(MyService.service, { sendMessage });
server.bindAsync('0.0.0.0:50051', grpc.ServerCredentials.createInsecure(), () => {
server.start();
console.log('Server running at http://0.0.0.0:50051');
});
}
main();
And On the Client Side:
const grpc = require('grpc');
const protoLoader = require('@grpc/proto-loader');
const PROTO_PATH = __dirname + '/path/to/proto/file.proto';
const packageDefinition = protoLoader.loadSync(PROTO_PATH);
const { MyService } = grpc.loadPackageDefinition(packageDefinition);
const client = new MyService('localhost:50051', grpc.credentials.createInsecure());
client.sendMessage({ content: 'Hello gRPC!' }, (error, response) => {
if (!error) {
console.log('Server Response:', response.reply);
} else {
console.error('Error:', error.message);
}
});
gRPC offers a compelling alternative to Websockets for real-time communication in Node.js applications, providing superior performance, strong typing, and bidirectional streaming capabilities. By following the steps outlined above, you can harness the power of gRPC to build efficient and scalable real-time systems that meet the demands of modern web applications.