Understanding Why React useState Doesn't Update in Window Events
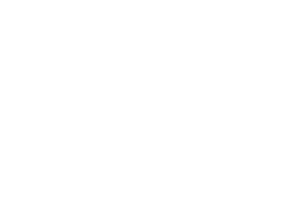
When working with React's useState hook, it's common to encounter issues with state updates within window event listeners. In this article, we'll explore three methods to address this problem and understand their inner workings.
1. Recreate and Re-register Handler on Each Change:
One way to ensure state updates within window event listeners is by recreating and re-registering the event handler every time the state changes. Here's how you can implement it:
const MyComponent = () => {
const [count, setCount] = useState(0);
useEffect(() => {
const handleResize = () => {
console.log(count); // Fresh count value
};
window.addEventListener('resize', handleResize);
return () => {
window.removeEventListener('resize', handleResize);
};
}, [count]);
return (
<div>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
};
2. Access Data by Reference:
Another approach involves accessing the state data by reference, ensuring event handlers have access to the updated state without causing re-renders:
const MyComponent = () => {
const countRef = useRef(0);
useEffect(() => {
const handleResize = () => {
console.log(countRef.current); // Access count via its ref
};
window.addEventListener('resize', handleResize);
return () => {
window.removeEventListener('resize', handleResize);
};
}, []);
const setCount = (newCount) => {
countRef.current = newCount;
};
return (
<div>
<button onClick={() => setCount(countRef.current + 1)}>Increment</button>
</div>
);
};
3. Use Functional Version of Setter to Access Most Recent Value:
The third method involves utilizing the functional version of the state setter provided by useState, ensuring event handlers access the most recent state value:
const MyComponent = () => {
const [count, setCount] = useState(0);
useEffect(() => {
const handleResize = () => {
setCount(prevCount => {
console.log(prevCount); // Access most recent count value
return prevCount;
});
};
window.addEventListener('resize', handleResize);
return () => {
window.removeEventListener('resize', handleResize);
};
}, []);
return (
<div>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
};
In conclusion, managing state updates within window event listeners in React requires thoughtful consideration. By employing one of these methods—recreating event handlers, accessing state by reference, or using the functional version of the setter—developers can ensure their components respond effectively to changes in both state and window events. The choice of method depends on the specific needs and constraints of the application.