High Cohesion in Software Development: Best Practices and Implementation Strategies
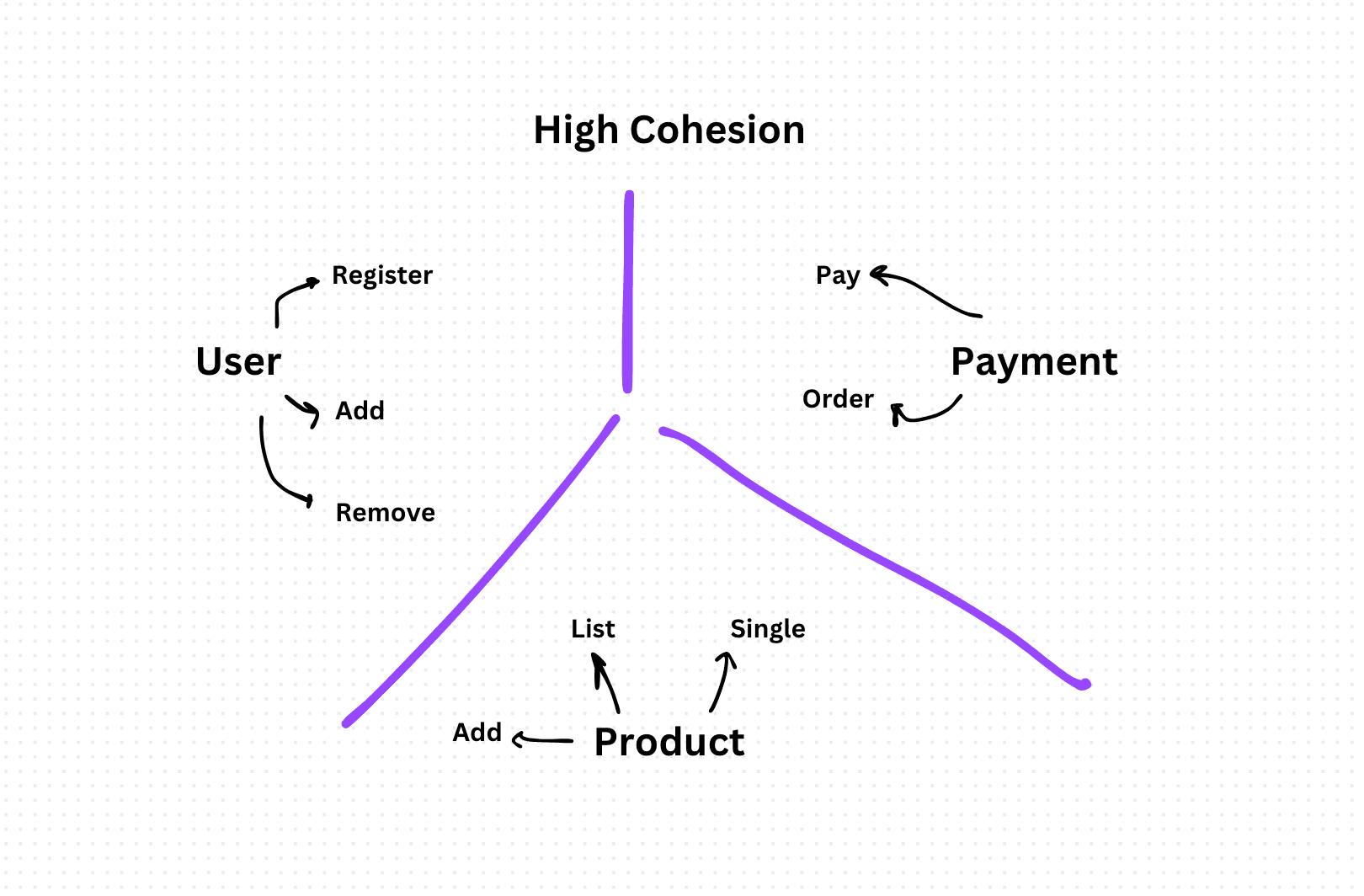
High cohesion refers to the degree to which elements within a module or component are related to each other and contribute to a single, well-defined purpose or responsibility. Modules with high cohesion have tightly focused functionality and are easier to understand, maintain, and reuse.
Benefits of High Cohesion:
- Improved Readability: High cohesion makes code easier to read and understand by grouping related functionality together, reducing cognitive load for developers.
- Enhanced Maintainability: Modules with high cohesion are easier to maintain and update because changes are localized to specific areas of the codebase.
- Increased Reusability: Cohesive modules are more reusable, as they encapsulate well-defined functionality that can be leveraged in different parts of the application or in other projects.
- Reduced Complexity: High cohesion reduces complexity by breaking down large, monolithic components into smaller, more manageable units with clear responsibilities.
- Better Testability: Cohesive modules are easier to test in isolation, leading to more comprehensive and targeted testing strategies.
Example: High Cohesion in TypeScript
class UserManager {
constructor(private userRepository: UserRepository, private logger: Logger) {}
addUser(user: User): void {
this.userRepository.save(user);
this.logger.log(`User added: ${user.name}`);
}
removeUser(userId: string): void {
const user = this.userRepository.findById(userId);
if (user) {
this.userRepository.delete(userId);
this.logger.log(`User removed: ${user.name}`);
} else {
this.logger.log(`User not found with ID: ${userId}`);
}
}
}
// Usage
const userRepository = new InMemoryUserRepository();
const logger = new ConsoleLogger();
const userManager = new UserManager(userRepository, logger);
userManager.addUser({ id: '1', name: 'John Doe' });
userManager.removeUser('1');
High cohesion is a key principle in software design that promotes readability, maintainability, and reusability of code. By following best practices such as adhering to the SRP, encapsulating related functionality, and modularizing code, developers can achieve high cohesion in their applications. Embracing high cohesion leads to cleaner, more maintainable codebases that are easier to understand, test, and evolve over time.