Best Practices for Writing Isolated Tests in TypeScript
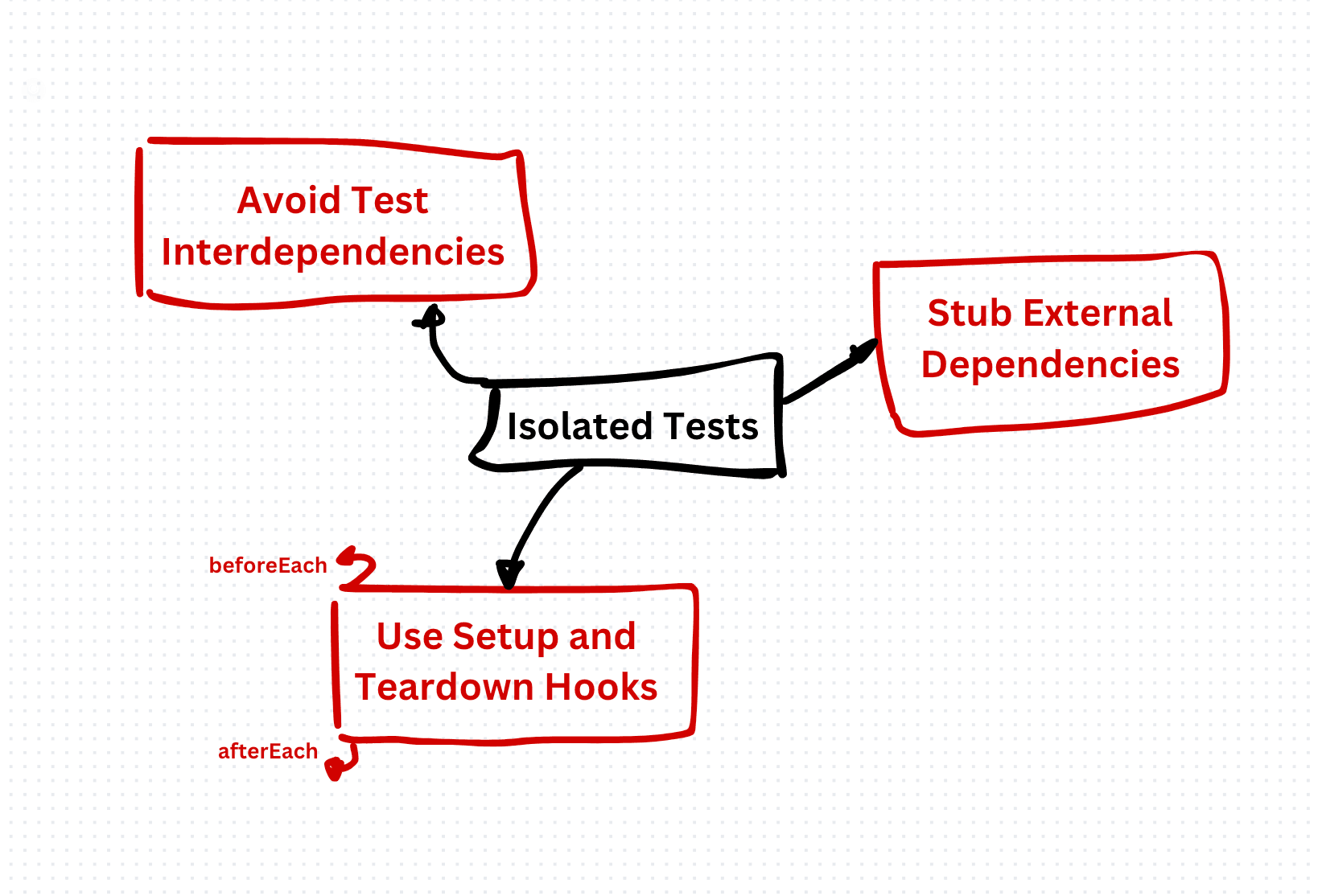
Isolation is a fundamental principle in testing, ensuring that each test case operates independently of others, thereby enhancing test reliability and maintainability. In this article, we'll explore best practices for writing isolated tests in TypeScript, along with examples demonstrating how to achieve test isolation effectively.
Key Best Practices:
- Avoid Test Interdependencies:
Tests should not rely on the state or outcome of other tests. Each test should set up its own context, execute the test scenario, and make assertions based solely on its own setup.
describe('Calculator', () => {
let calculator: Calculator;
beforeEach(() => {
calculator = new Calculator();
});
it('should add two numbers correctly', () => {
const result = calculator.add(2, 3);
expect(result).toBe(5);
});
it('should subtract two numbers correctly', () => {
const result = calculator.subtract(5, 2);
expect(result).toBe(3);
});
});
- Use Setup and Teardown Hooks:
Leverage setup (beforeEach) and teardown (afterEach) hooks to establish a clean state before each test runs and to clean up any resources afterward. This ensures that tests start and end in a predictable state, regardless of the order of execution.
describe('UserService', () => {
let userService: UserService;
let databaseMock: Database;
beforeEach(() => {
databaseMock = createDatabaseMock();
userService = new UserService(databaseMock);
});
it('should retrieve a user by ID from the database', () => {
});
afterEach(() => {
databaseMock.close(); // Clean up resources
});
});
- Stub External Dependencies:
Replace real dependencies with test doubles such as stubs, mocks, or fakes to isolate the code under test from external influences. This allows tests to focus solely on the behavior of the unit being tested.
describe('UserService', () => {
let userService: UserService;
let databaseStub: DatabaseStub;
beforeEach(() => {
databaseStub = createDatabaseStub();
userService = new UserService(databaseStub);
});
it('should retrieve a user by ID from the database', () => {
databaseStub.getUserById.returns({ id: '123', name: 'John' });
const user = userService.getUserById('123');
expect(user.name).toBe('John');
});
});
By adhering to best practices for writing isolated tests in TypeScript, developers can create test suites that are robust, reliable, and maintainable. Avoiding test interdependencies, using setup and teardown hooks effectively, and stubbing external dependencies enable tests to focus on individual units of code, facilitating faster feedback and easier debugging.