Secure Programming and Resilience (SPR) Best Practices in TypeScript
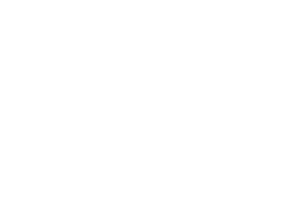
Secure Programming and Resilience (SPR) refers to a set of principles and practices aimed at developing robust, secure, and resilient software systems. In TypeScript development, adhering to SPR best practices is crucial for building applications that can withstand security threats, failures, and unexpected events.
Key SPR Best Practices:
- Input Validation:
Ensure that all user input and external data are validated and sanitized to prevent injection attacks, such as SQL injection, XSS, and CSRF. Use TypeScript's type system and validation libraries to enforce data integrity and protect against malicious input.Example:
function validateEmail(email: string): boolean {
return /^[^\s@]+@[^\s@]+\.[^\s@]+$/.test(email);
}
- Error Handling:
Implement robust error handling mechanisms to gracefully handle exceptions, errors, and failures. Use TypeScript's try-catch blocks, error objects, and custom error classes to capture and handle errors effectively, providing informative error messages and logging for debugging and troubleshooting.Example:
try {
} catch (error) {
console.error('An error occurred:', error.message);
}
- Authentication and Authorization:
Secure endpoints and resources with proper authentication and authorization mechanisms. Use TypeScript frameworks like Express.js with middleware such as Passport.js for implementing authentication strategies like JWT, OAuth, or session-based authentication, and enforce access control based on user roles and permissions
function authenticate(req, res, next) {
if (req.isAuthenticated()) {
return next();
}
res.status(401).json({ message: 'Unauthorized' });
}
- Data Encryption:
Protect sensitive data by encrypting it at rest and in transit. Use TypeScript libraries like crypto-js for symmetric and asymmetric encryption, and TLS/SSL for secure communication over HTTPS.
import CryptoJS from 'crypto-js';
const encryptedData = CryptoJS.AES.encrypt('secretData', 'secretKey').toString();
- Resilient Architecture:
Design applications with resilience in mind to handle failures and recover gracefully. Implement fault tolerance mechanisms such as circuit breakers, retries, and timeouts to mitigate cascading failures and ensure system availability and performance under adverse conditions.
function fetchData(url: string, retries: number = 3): Promise<any> {
return fetch(url)
.then(response => response.json())
.catch(error => {
if (retries > 0) {
console.warn('Retrying...');
return fetchData(url, retries - 1);
}
throw new Error('Failed to fetch data');
});
}
By following SPR best practices in TypeScript development, developers can build secure, resilient, and high-quality software systems that protect against security threats, handle errors gracefully, and maintain performance and availability in the face of failures. By incorporating input validation, error handling, authentication and authorization, data encryption, and resilient architecture into their applications, developers can create robust solutions that meet the highest standards of security and reliability.