Hash Tables Data Structure in TypeScript: A Comprehensive Guide
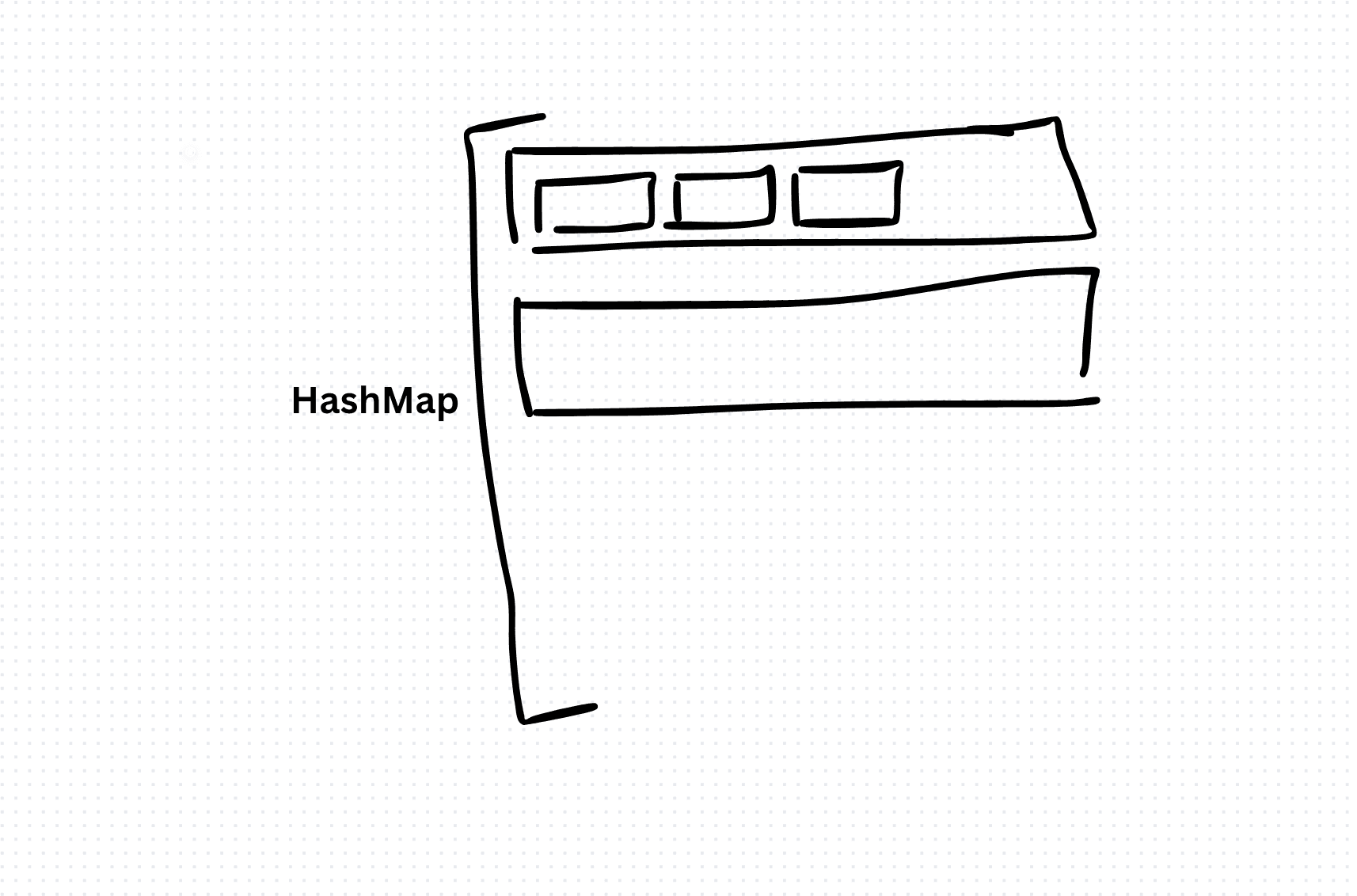
A hash table is a data structure that stores key-value pairs, where each key is mapped to a unique index using a hashing function. This mapping allows for constant-time average-case performance for insertion, deletion, and retrieval operations.
Implementation in TypeScript:
Let's explore the implementation of hash tables in TypeScript with practical examples:
class HashTable<K, V> {
private table: Map<number, V>[]; // Array of Maps
constructor(size: number) {
this.table = new Array(size).fill(null).map(() => new Map());
}
private hash(key: K): number {
return String(key).length % this.table.length;
}
insert(key: K, value: V): void {
const index = this.hash(key);
this.table[index].set(key, value);
}
get(key: K): V | undefined {
const index = this.hash(key);
return this.table[index].get(key);
}
remove(key: K): void {
const index = this.hash(key);
this.table[index].delete(key);
}
}
const hashTable = new HashTable<string, number>(10);
hashTable.insert("apple", 5);
hashTable.insert("banana", 8);
console.log(hashTable.get("apple")); // Output: 5
hashTable.remove("banana");
console.log(hashTable.get("banana"));
When to use Hash Tables?
- Fast Lookup: HashTables provide constant-time (O(1)) average-case lookup complexity, making them ideal for applications requiring fast data retrieval based on keys.
- Associative Arrays: HashTables are often used to implement associative arrays, where each key is associated with a value. This makes them suitable for tasks like caching, indexing, and storing configuration data.
- Data Indexing: In databases and search engines, HashTables are used to index data efficiently. They enable quick lookups based on keys, allowing for faster query processing and data retrieval.
- Implementing Caches: HashTables are employed in caching mechanisms to store frequently accessed data. Cached data can be quickly retrieved based on keys without having to perform expensive computations or I/O operations.
- Symbol Tables in Compilers: HashTables are used to implement symbol tables in compilers and interpreters. They facilitate fast lookup of identifiers (e.g., variables, functions) during lexical analysis and parsing stages.
- Storing Unique Values: HashTables can be utilized to store unique values efficiently. By using keys as unique identifiers, HashTables prevent duplicate entries and enable quick checks for value existence.
- Implementing Hash-Based Data Structures: HashTables serve as fundamental components in implementing other data structures like HashMaps, HashSet, and LinkedHashMaps. These data structures leverage HashTables to achieve fast key-based operations.
Hash tables are versatile data structures that offer efficient storage and retrieval of key-value pairs. By leveraging hashing functions and collision resolution techniques, hash tables provide constant-time average-case performance for essential operations. Whether you're implementing a caching mechanism, indexing system, or associative array, hash tables in TypeScript offer a reliable and scalable solution for managing key-value data. With a solid understanding of hash tables and their operations, you can tackle a wide range of programming challenges with confidence.
More: