Optimizing Database Queries: Techniques and Best Practices
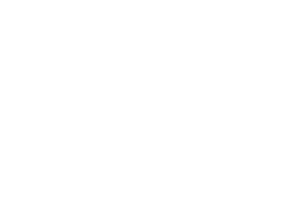
Efficient database query optimization is crucial for improving application performance, reducing response times, and minimizing resource consumption. In this technical article, we'll explore various methods and best practices to optimize database queries, focusing on techniques applicable to both SQL and NoSQL databases. We'll discuss indexing, query tuning, caching, denormalization, and other strategies with practical examples to illustrate their impact on query performance.
Method 1: Indexing
Indexing plays a crucial role in optimizing query performance by enabling rapid data retrieval based on specific criteria. Indexes provide efficient access paths to data, reducing the need for full-table scans and speeding up query execution. Common types of indexes include:
- B-tree Index: Suitable for range queries and equality predicates.
- Hash Index: Ideal for exact match queries.
- Full-Text Index: Used for searching text data efficiently.
CREATE INDEX idx_last_name ON employees (last_name);
This SQL statement creates an index on the "last_name" column of the "employees" table, improving performance for queries that filter or sort by last name.
Method 2: Query Tuning
Query tuning involves optimizing SQL queries to improve execution plans, minimize resource consumption, and reduce query execution times. Techniques for query tuning include:
- Selective Projection: Retrieve only the required columns to minimize data transfer.
- Predicate Pushdown: Apply filters as early as possible in the query execution process.
- Join Optimization: Choose optimal join algorithms and join order to minimize processing overhead.
SELECT employee_id, first_name, last_name
FROM employees
WHERE department_id = 10;
This SQL query selects specific columns and applies a filter predicate to reduce the amount of data processed by the database engine.
Method 3: Caching:
Caching involves storing frequently accessed query results or data in memory to reduce latency and improve response times. By caching query results, applications can avoid redundant database accesses for repetitive queries, improving overall performance. Example (Redis Cache):
const redis = require('redis');
const client = redis.createClient();
app.get('/employees/:id', (req, res) => {
const employeeId = req.params.id;
client.get(`employee:${employeeId}`, (err, reply) => {
if (reply) {
// Return cached data
res.json(JSON.parse(reply));
} else {
// Query database and cache result
Employee.findById(employeeId, (err, employee) => {
client.setex(`employee:${employeeId}`, 3600, JSON.stringify(employee));
res.json(employee);
});
}
});
});
Method 4: Denormalization:
Denormalization involves storing redundant or precomputed data in the database to optimize query performance at the expense of data redundancy. By reducing the need for complex joins and aggregations, denormalization can improve query execution times for read-heavy workloads.
CREATE TABLE employee_summary (
department_id INT,
avg_salary DECIMAL(10, 2),
max_salary DECIMAL(10, 2),
min_salary DECIMAL(10, 2),
PRIMARY KEY (department_id)
);
This denormalized table stores summarized salary information for each department, eliminating the need for costly aggregations when querying employee salary statistics.
Optimizing database queries is essential for enhancing application performance and scalability. By employing techniques such as indexing, query tuning, caching, and denormalization, developers can minimize query execution times, reduce resource utilization, and deliver responsive user experiences. Understanding the principles and best practices of database query optimization empowers developers to build efficient and high-performing applications in any database environment.