Bridge Design Pattern
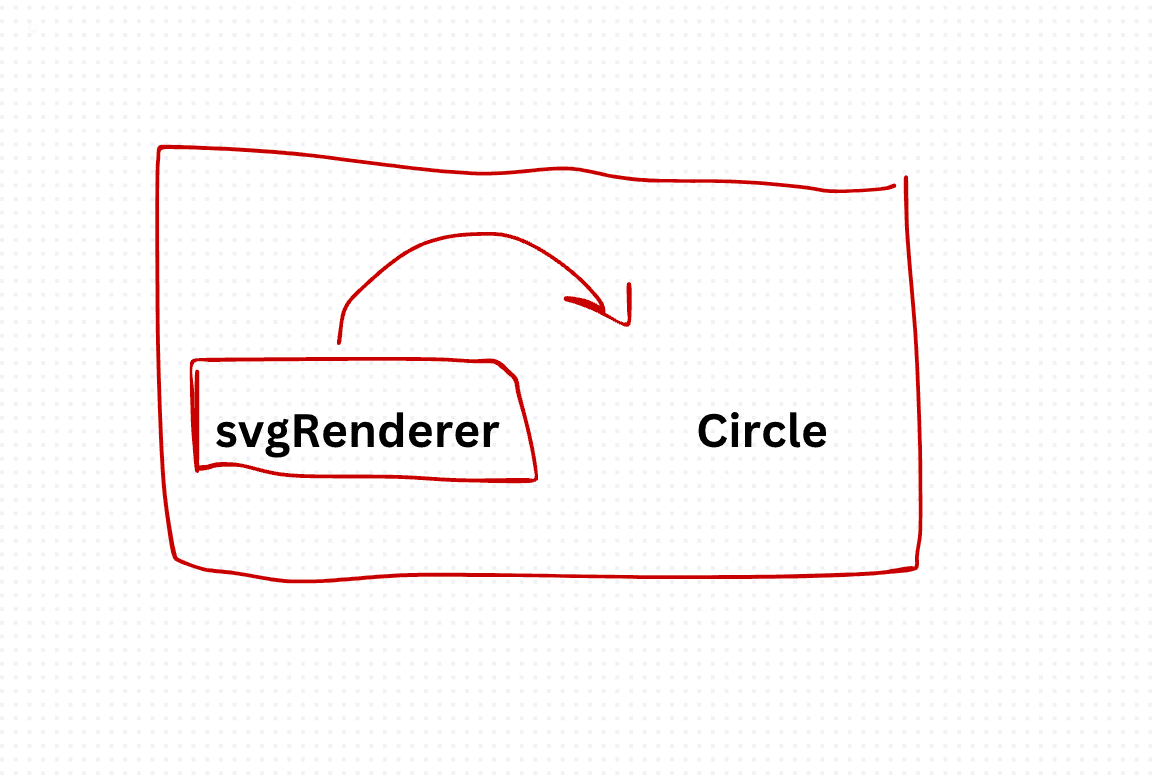
At its core, the Bridge Design Pattern promotes flexibility and extensibility by separating the abstraction (i.e., high-level logic) from its implementation (i.e., low-level details). By encapsulating these concerns in separate hierarchies, the pattern allows them to evolve independently, facilitating easier maintenance and scalability.
// Implementor interface
class Renderer {
renderCircle(radius) {
throw new Error("renderCircle method must be implemented.");
}
}
// Concrete Implementor: SVG Renderer
class SvgRenderer extends Renderer {
renderCircle(radius) {
console.log(`Drawing circle with radius ${radius} using SVG.`);
}
}
// Concrete Implementor: Canvas Renderer
class CanvasRenderer extends Renderer {
renderCircle(radius) {
console.log(`Drawing circle with radius ${radius} using Canvas.`);
}
}
// Abstraction
class Shape {
constructor(renderer) {
this.renderer = renderer;
}
draw() {
throw new Error("draw method must be implemented.");
}
}
// Refined Abstraction: Circle
class Circle extends Shape {
constructor(renderer, radius) {
super(renderer);
this.radius = radius;
}
draw() {
this.renderer.renderCircle(this.radius);
}
}
// Usage
const svgRenderer = new SvgRenderer();
const circle1 = new Circle(svgRenderer, 5);
circle1.draw(); // Output: Drawing circle with radius 5 using SVG.
const canvasRenderer = new CanvasRenderer();
const circle2 = new Circle(canvasRenderer, 10);
circle2.draw();
The Bridge Design Pattern promotes loose coupling and flexibility by separating abstraction from implementation. By employing this pattern, developers can create hierarchies of related classes that can evolve independently, leading to more maintainable and extensible codebases.