Container Component React Design Pattern
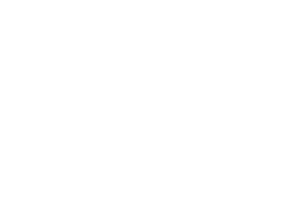
Let's break it down in simple terms. The Container Component Pattern is like having two types of superheroes in your React app.
At its core, the Container Component Pattern emphasizes the separation of concerns by distinguishing between two types of components: container components and presentational components.
Principles of Container Component Pattern:
- Single Responsibility Principle (SRP): Container components adhere to the SRP by focusing on a specific aspect of application logic, such as managing state or handling data fetching, thus promoting modular and maintainable code.
- Separation of Concerns: By separating state management and UI rendering, the Container Component Pattern facilitates code organization and enhances code reusability, making it easier to reason about and maintain.
- Data Flow Control: Container components serve as the bridge between the application's data layer and the UI components, enabling controlled data flow and facilitating communication between different parts of the application.
For Example:
// Container Component (UserContainer.js)
import React, { useState, useEffect } from 'react';
import UserDataService from './services/UserDataService'; // Assume this exists
const UserContainer = () => {
const [users, setUsers] = useState([]);
useEffect(() => {
const fetchUsers = async () => {
try {
const response = await UserDataService.getUsers();
setUsers(response.data);
} catch (error) {
console.error('Error fetching users:', error);
}
};
fetchUsers();
}, []);
return (
<div>
<h2>User List</h2>
<ul>
{users.map(user => (
<li key={user.id}>{user.name}</li>
))}
</ul>
</div>
);
};
export default UserContainer;
In this example, UserContainer is our container component responsible for fetching user data from an API and managing the state of the user list.
And Presentation Component will be like:
import React from 'react';
const UserList = ({ users }) => (
<div>
<h2>User List</h2>
<ul>
{users.map(user => (
<li key={user.id}>{user.name}</li>
))}
</ul>
</div>
);
export default UserList;
There you have it – the Container Component Pattern in all its glory! By letting container components handle the heavy lifting and presentational components handle the UI dazzle, you'll create React apps that are clean, maintainable, and downright awesome. So go ahead, give it a whirl in your next React project, and watch your app shine! ✨