Context API React Design Pattern
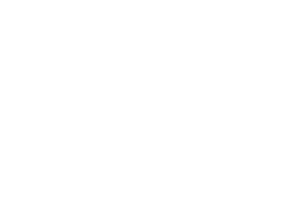
The Context API is like a hidden treasure chest buried deep within the React ecosystem. It provides a way to share data between components without having to pass props down through every level of the component tree. Think of it as a global messenger that delivers data to any component that needs it, no matter how deep it is in the tree.
What sets the Context API apart is its simplicity and power. With just a few lines of code, you can create a centralized store for your application's state and access it from any component. This eliminates the need for prop drilling and makes your code more concise and maintainable.
Implementing the Context API Design Pattern:
Let's dive into a simple example to see the Context API in action:
import { createContext, useState } from 'react';
const ThemeContext = createContext();
export const ThemeProvider = ({ children }) => {
const [theme, setTheme] = useState('light');
const toggleTheme = () => {
setTheme((prevTheme) => (prevTheme === 'light' ? 'dark' : 'light'));
};
return (
<ThemeContext.Provider value={{ theme, toggleTheme }}>
{children}
</ThemeContext.Provider>
);
};
export default ThemeContext;
In this example, we create a ThemeProvider component that wraps our entire application and provides a theme and toggleTheme function via the ThemeContext.Provider.
import React from 'react';
import { ThemeProvider } from './ThemeContext';
import Header from './Header';
import Content from './Content';
const App = () => (
<ThemeProvider>
<Header />
<Content />
</ThemeProvider>
);
export default App;
Meanwhile, in our App component, we wrap our header and content components with the ThemeProvider to give them access to the theme context.
And there you have it – the Context API Design Pattern demystified! With the power of context, you can streamline state management in your React applications and build more maintainable and scalable codebases. So go ahead, dive into the world of context, and unlock new possibilities for your React projects