Best Practice FrontEnd React App Design Pattern
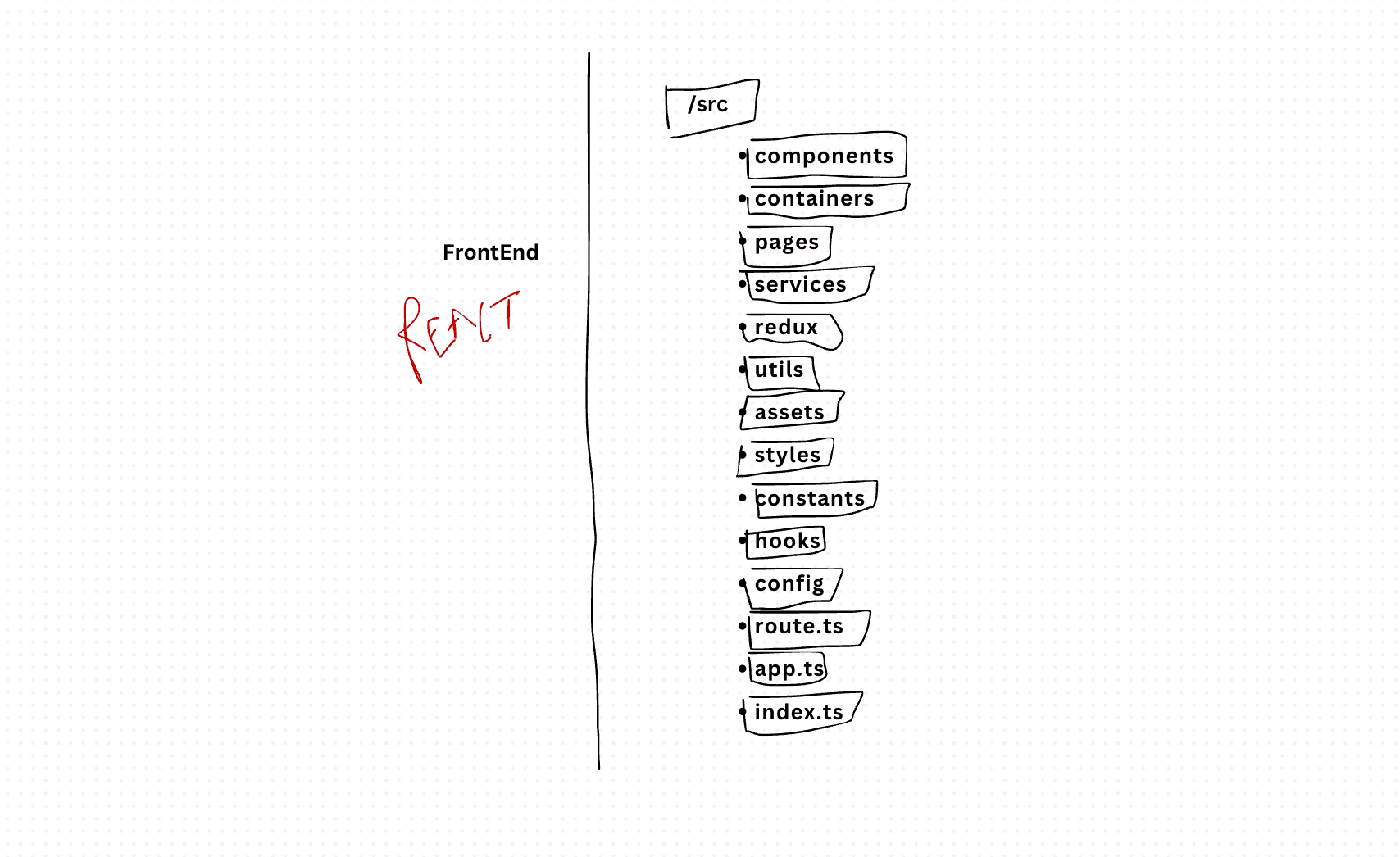
Designing a robust and scalable frontend architecture in React involves organizing your codebase in a structured manner to promote maintainability, reusability, and scalability. Here's a suggested architecture for a React application:
Folder Structure in /src:
- components/: Contains reusable UI components.
- containers/: Components that connect to Redux (or other state management libraries) and fetch data from APIs.
- pages/: Top-level components representing different pages/routes of your application.
- services/: Utility functions for making API calls, handling authentication, etc.
- redux/: Contains Redux-related files such as actions, reducers, and store configuration.
- utils/: Utility functions used across the application.
- assets/: Images, fonts, and other static assets.
- styles/: Global styles, SCSS variables, mixins, etc.
- constants/: Constants used across the application.
- hooks/: Custom React hooks.
- config/: Configuration files for environment variables, API endpoints, etc.
- routes.ts: Defines the application routes.
- App.ts: Main component where routing and global layout are defined.
- index.ts: Entry point of the application.
Principles to follow:
- Each component should ideally be self-contained and reusable.
- Follow the principles of separation of concerns, keeping components focused on specific tasks.
- Use functional components with hooks for state management where possible, unless you need lifecycle methods or local state.
- Utilize Redux, MobX, or React Context API for managing application state, depending on the complexity of your application.
- Separate your state into slices/modules to ensure modularity and maintainability.
- Use Redux middleware for async actions (e.g., Redux Thunk or Redux Saga).
- Encapsulate API calls within service/utility functions.
- Use axios or fetch for making HTTP requests.
- Implement error handling and data normalization within API services.
- Use React Router for declarative routing.
- Define routes in a separate file (e.g., routes.js) for better organization.
- Utilize CSS modules, styled-components, or another CSS-in-JS solution for styling components.
- Maintain consistency by using a design system or component library.
- Write unit tests for components, containers, and utility functions using Jest and React Testing Library.
- Implement end-to-end testing using tools like Cypress or Selenium for critical user flows.
- Ensure that your application is accessible by following best practices such as providing semantic HTML, keyboard navigation, and proper ARIA attributes.
- Implement i18n and l10n early in your project to support multiple languages and locales.
- Implement error boundaries to catch errors and prevent them from crashing the entire application.
- Integrate logging solutions such as Sentry or LogRocket to track errors and debug issues in production.
- Set up a CI/CD pipeline for automated testing and deployment.
- Deploy your application to a cloud platform like AWS, Azure, or Netlify.
- Optimize bundle size by code splitting and lazy loading components.
- Utilize memoization and useMemo/useCallback hooks to optimize rendering performance.
- Implement server-side rendering (SSR) or static site generation (SSG) for improved SEO and initial load times.
By following these guidelines, you can create a well-structured and maintainable React application that is scalable and easy to work with as your project grows.
In summary, adopting a well-structured folder layout is crucial for maintaining a clean, organized, and scalable codebase in a React application. By following best practices and design patterns, developers can streamline development workflows, improve code maintainability, and enhance collaboration among team members.