Prototype Design Pattern
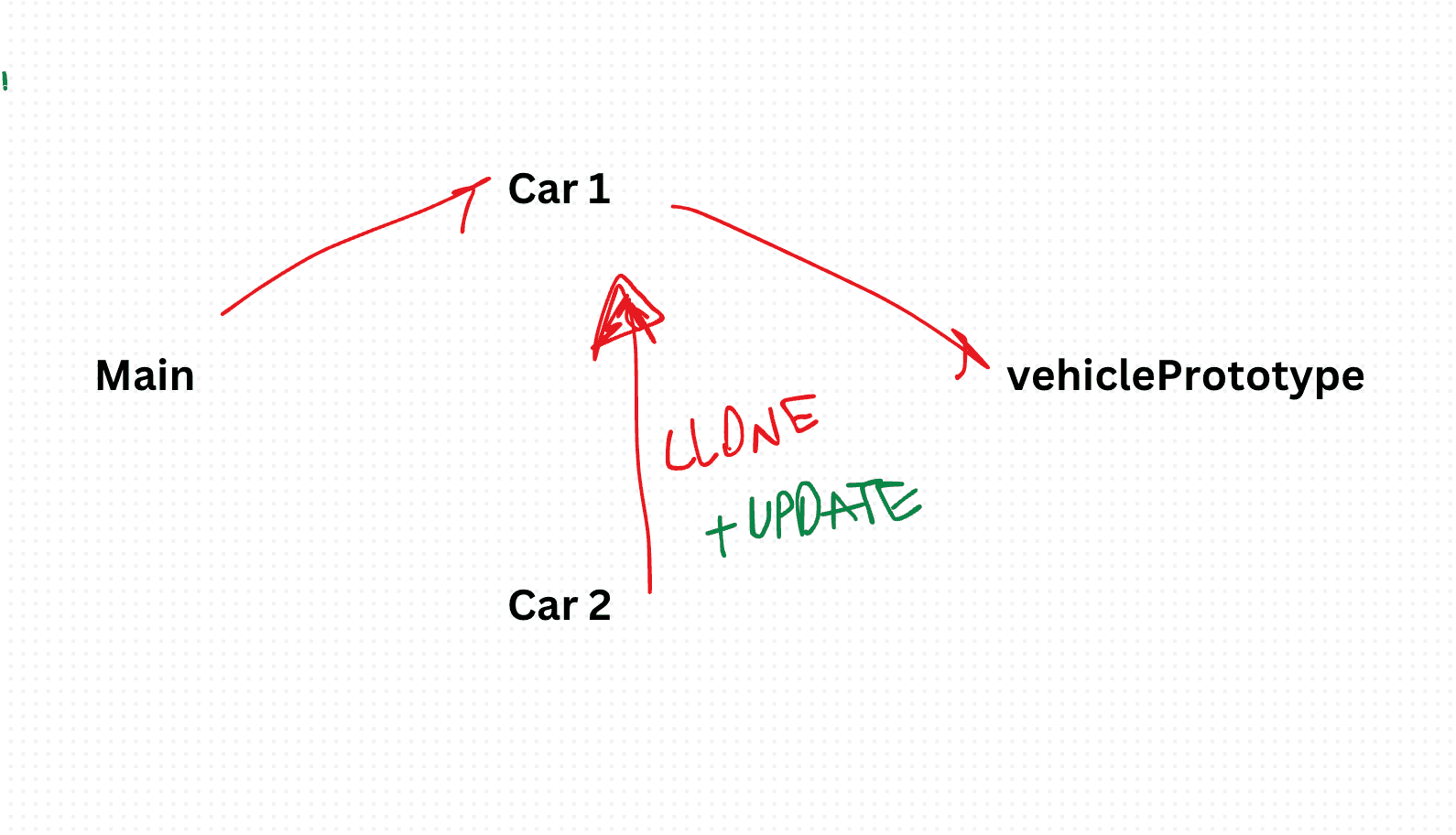
The Prototype Design Pattern promotes flexibility and efficiency in object creation by leveraging prototypical inheritance. Instead of creating new instances of objects from scratch, we can clone an existing prototype object and modify its properties as needed.
const vehiclePrototype = {
type: "car",
make: "Toyota",
model: "Camry",
year: 2022,
clone() {
return Object.create(this);
}
};
const vehicle1 = vehiclePrototype.clone();
const vehicle2 = vehiclePrototype.clone();
vehicle1.model = "Corolla";
vehicle2.year = 2023;
console.log(vehicle1); // Output: { type: 'car', make: 'Toyota', model: 'Corolla', year: 2022 }
Cloned objects are created using the clone method, which returns a shallow copy of the prototype object using Object.create(this).
The Prototype Design Pattern offers a flexible and efficient way to create new objects by cloning existing prototype instances. By leveraging prototypical inheritance, developers can avoid the overhead of creating new objects from scratch and achieve greater code reusability and performance.