Thread Pool Design Pattern
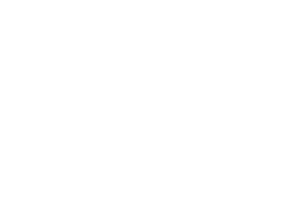
The Thread Pool Design Pattern involves creating a pool of worker threads that are managed and reused to execute tasks concurrently. Instead of creating and destroying threads for each task, a fixed number of threads are initialized upfront and kept alive throughout the application's lifecycle. Tasks are submitted to the thread pool, and available threads execute them asynchronously. This pattern helps avoid the overhead of thread creation and termination, leading to improved performance and scalability.
class ThreadPool {
constructor(size) {
this.size = size;
this.workers = Array.from({ length: size }, () => new Worker('worker.js'));
this.availableWorkers = [...this.workers];
}
executeTask(task) {
if (this.availableWorkers.length === 0) {
throw new Error('No available workers in the thread pool');
}
const worker = this.availableWorkers.pop();
worker.postMessage(task);
worker.onmessage = () => {
this.availableWorkers.push(worker);
};
}
}
// Example usage
const threadPool = new ThreadPool(4);
function taskFunction(data) {
console.log('Task executed with data:', data);
}
threadPool.executeTask({ task: taskFunction, data: 'Task 1' });
threadPool.executeTask({ task: taskFunction, data: 'Task 2' });
The Thread Pool Design Pattern offers a practical solution for managing threads efficiently in JavaScript applications. By pre-initializing a pool of worker threads and reusing them to execute tasks concurrently, this pattern reduces the overhead of thread creation and termination, leading to improved performance and resource utilization. In JavaScript, the Thread Pool pattern can be implemented using the Worker API or other concurrency mechanisms provided by modern web browsers and Node.js environments.