What is Cross-Site Scripting (XSS), and how can it be prevented?
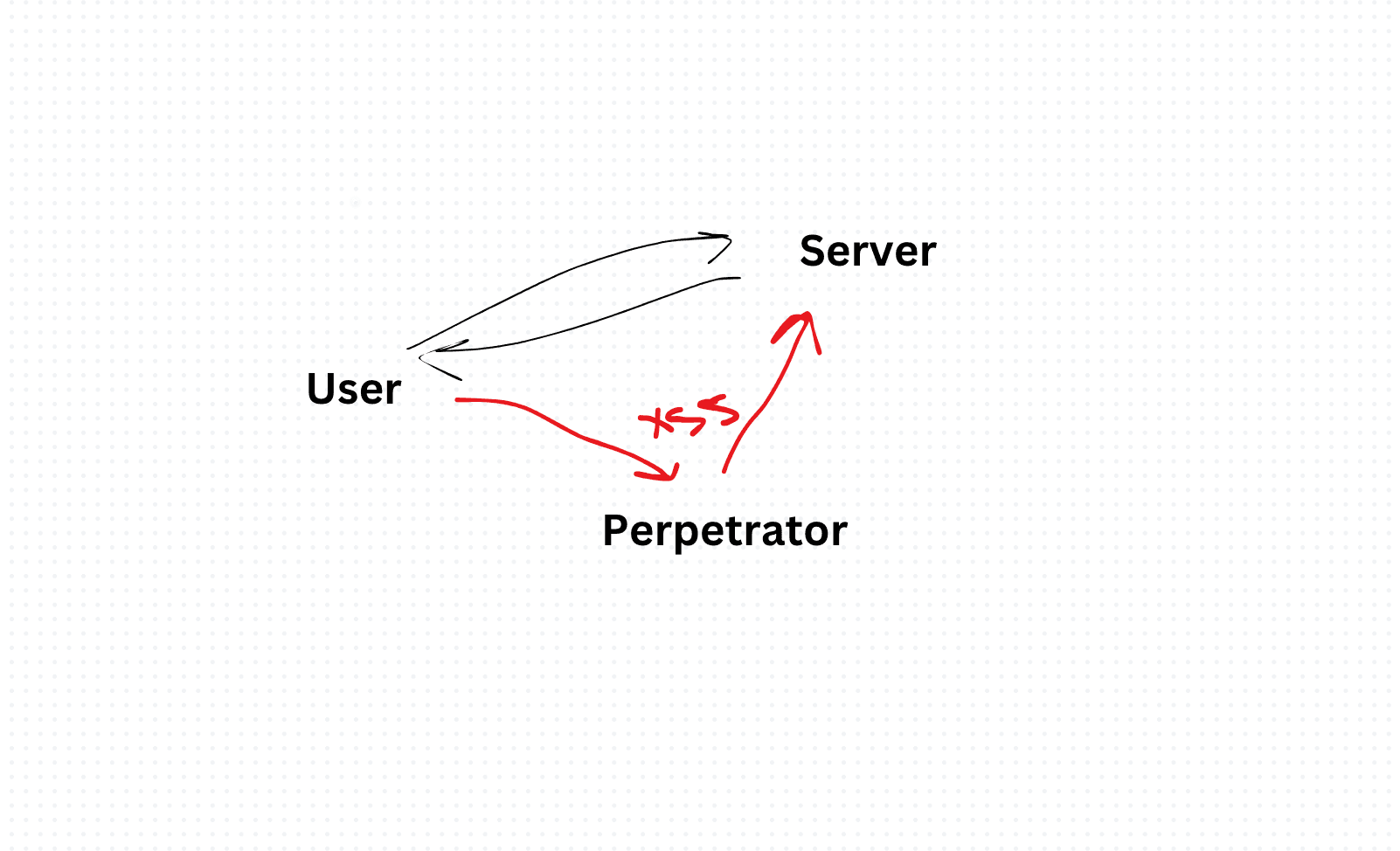
XSS is a notorious security vulnerability where attackers inject malicious scripts into web pages, putting users' data and privacy at risk. Today, we'll understand how XSS works and explore some nifty JavaScript techniques to prevent it.
Understanding Cross-Site Scripting (XSS):
So, here's the deal with XSS: attackers slip in malicious scripts, often JavaScript, into web pages. When innocent users visit these pages, the malicious scripts get executed in their browsers, opening the floodgates for all sorts of mayhem like data theft and session hijacking.
- Reflected XSS: The injected script bounces off a server and runs in the victim's browser.
- Stored XSS: The injected script sticks around on the server, waiting to pounce on unsuspecting users.
- DOM-based XSS: This one's tricky; attackers mess with the Document Object Model (DOM) of a web page, setting up traps for users.
Preventing XSS with JavaScript:
Now, let's get to the good stuff – how to stop XSS dead in its tracks using JavaScript.
- Input Validation: Scrutinize and sanitize user input like your life depends on it. Make sure no sneaky scripts slip through the cracks.
function sanitizeInput(input) {
return input.replace(/<[^>]*>?/gm, '');
}
- Escaping Output: Before displaying user-generated content, give it a good scrubbing. Encode it so that it's harmless to our precious web page.
function escapeHTML(html) {
return document.createElement('div').appendChild(document.createTextNode(html)).parentNode.innerHTML;
}
- Content Security Policy (CSP): Set up some ground rules for your web server. With CSP headers, you can dictate what content is allowed on your web page, leaving no room for XSS shenanigans.
res.setHeader('Content-Security-Policy', "script-src 'self'");
- HTTPOnly Cookies: Lock down your cookies so that pesky scripts can't get their grubby hands on them. Set the HTTPOnly flag to keep them safe.
res.cookie('session', '123456', { httpOnly: true });
- DOM Manipulation: When messing with the DOM, stick to safe methods like textContent. Don't leave any cracks for XSS to sneak through.
element.textContent = userContent;
Cross-Site Scripting (XSS) may sound intimidating, but armed with the right knowledge and techniques, we can keep our web applications safe and secure. By validating inputs, escaping outputs, and enforcing strict security policies, we can thwart XSS attacks and ensure the safety of our users' data.