Testing React Components in Storybook Using Testing Library
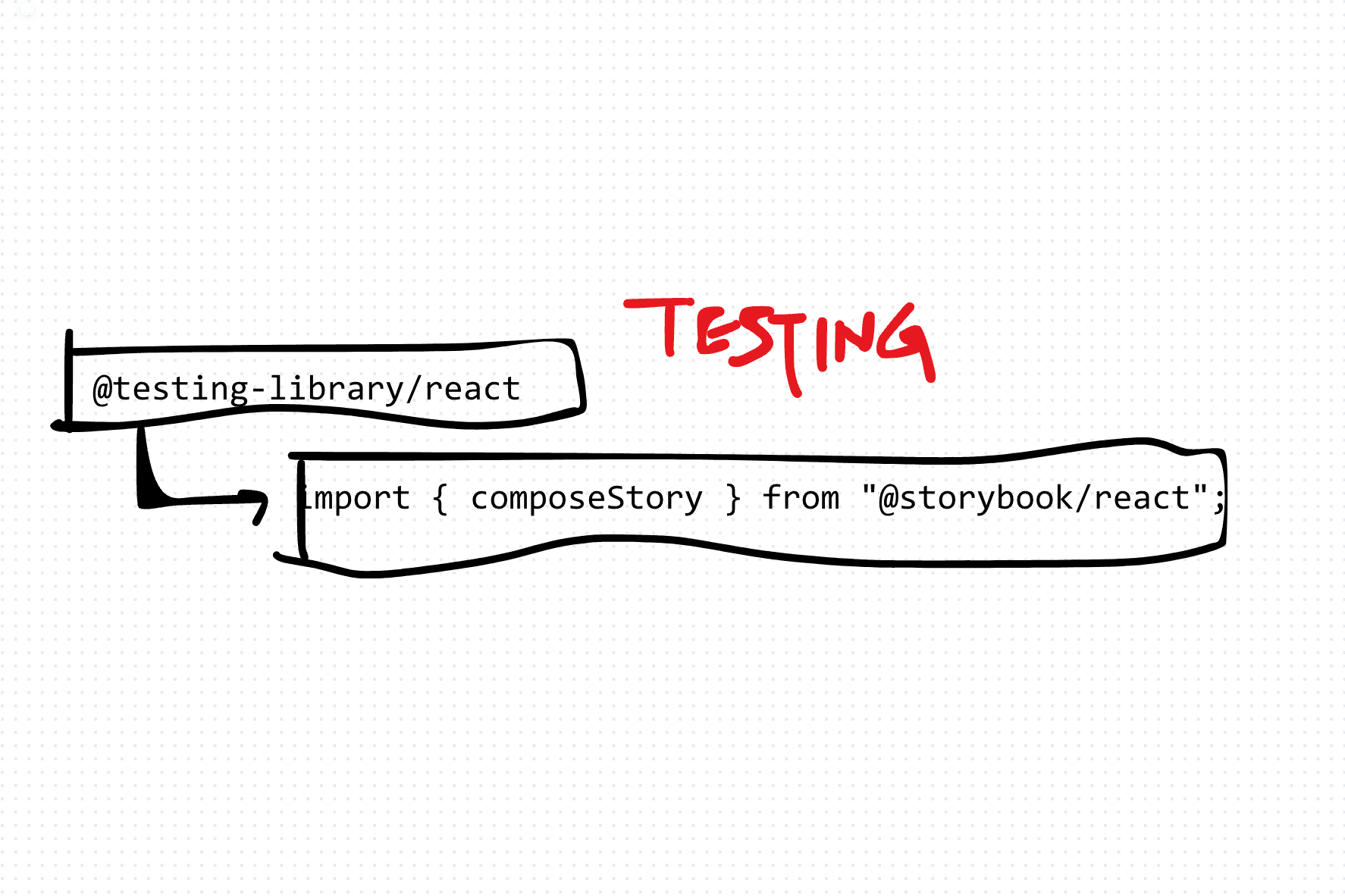
Testing React components in isolation within a Storybook environment offers several advantages, including the ability to test component variations and edge cases efficiently. By using Testing Library alongside Storybook, developers can write tests that accurately reflect user interactions and validate component behavior across different scenarios.
Testing a Button Component
Let's dive into testing a button component within Storybook using Testing Library. We'll cover various aspects of testing, including verifying button functionality, testing component themes, handling click actions, and more.
- Verifying Button Functionality
The first step is to ensure that the button component renders correctly with the specified text content. We can use the getByText query from Testing Library to select the button element and assert its presence.
import { fireEvent, render, screen } from "@testing-library/react";
import { composeStory } from "@storybook/react";
import Meta, {
Primary,
PrimaryWithIcon,
PrimaryWithCaret,
PrimaryDisabled,
PrimaryDisabledWithIcon,
PrimaryDisabledWithCaret,
PrimaryHover,
PrimaryHoverWithIcon,
PrimaryHoverWithCaret,
Secondary,
} from "./Button.stories";
const PrimaryStory = composeStory(Primary, Meta);
test("Button children to work", () => {
render(<PrimaryStory>Button</PrimaryStory>);
const buttonElement = screen.getByText("Button");
expect(buttonElement).toBeTruthy();
});
- Testing Component Themes
Next, we'll test the button component's theme variants, such as primary and secondary themes. We can use the getByTestId query to select the button element and assert the presence of specific CSS classes representing different themes.
test("Button to have primary theme", () => {
render(<PrimaryStory dataTestId="button">Button</PrimaryStory>);
const buttonElement = screen.getByTestId("button");
expect(buttonElement.classList).toContain("button--primary");
});
- Handling Click Actions
To test the click action of the button component, we'll simulate a user click using the fireEvent.click method from Testing Library. We'll also mock a click handler function using jest.fn() to ensure that it gets called when the button is clicked.
test("Primary click action to work", () => {
const handleClick = jest.fn();
render(
<PrimaryStory onClick={handleClick} dataTestId="button">
Button
</PrimaryStory>
);
const buttonElement = screen.getByTestId("button");
fireEvent.click(buttonElement);
expect(handleClick).toHaveBeenCalledTimes(1);
});
Testing React components within Storybook using Testing Library offers a powerful way to ensure component functionality and behavior meet the expected standards. By following the outlined testing approach and leveraging Testing Library's queries and utilities, developers can create robust and maintainable tests for their React components in Storybook environments.