ReturnType, Parameters, and Readonly in TypeScript
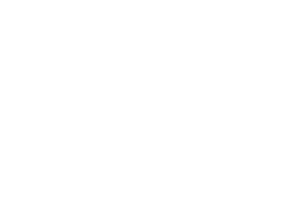
ReturnType is a type that represents the return type of a function. Parameters is a type that represents the parameters of a function. Readonly is a type that represents a type that is read-only.
function greet(name: string): string {
return `Hello, ${name}!`;
}
type GreetReturnType = ReturnType<typeof greet>;
The GreetReturnType type will be a type that represents the return type of the greet function, which is string.
type GreetParameters = Parameters<typeof greet>;
The GreetParameters type will be a type that represents the parameters of the greet function, which is string.
type ReadonlyString = Readonly<string>;
The ReadonlyString type will be a type that represents a read-only string.
ReturnType, Parameters, and Readonly are powerful types in TypeScript that can be used to create powerful type guards that enforce the structure of an object. By using these types, you can improve the type safety, maintainability, and reusability of your code. In this article, we've seen how to use ReturnType, Parameters, and Readonly to create type guards that enforce the structure of an object. With these types, you can write more robust and maintainable code.