TypeScript Casting: A Powerful Tool for Type Safety
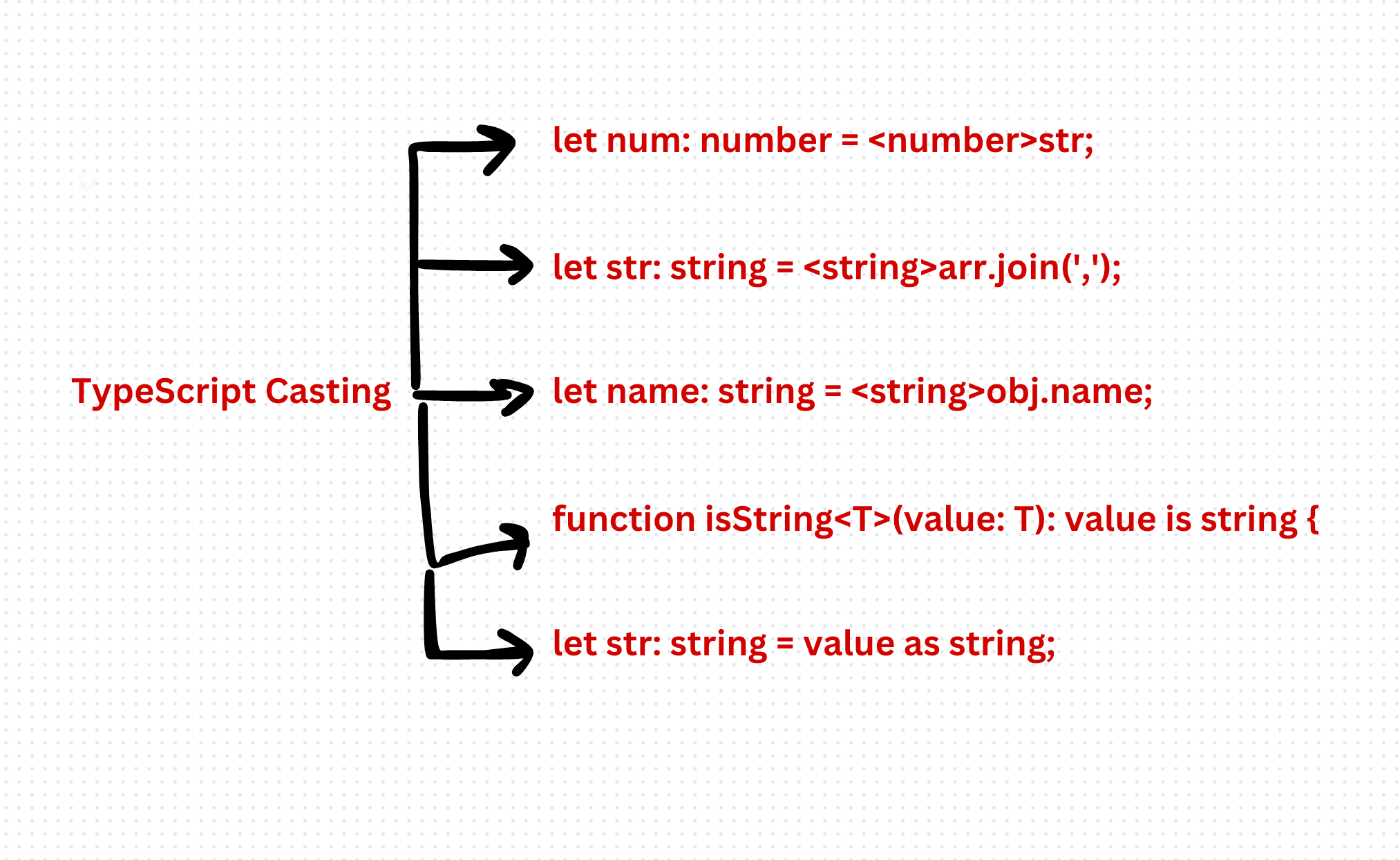
As a developer, you're likely familiar with the importance of type safety in your code. TypeScript is a statically-typed language that helps you catch type-related errors at compile-time, making it easier to write maintainable and scalable code. One of the most powerful tools in TypeScript is casting, which allows you to explicitly convert a value from one type to another.
TypeScript casting is a way to explicitly convert a value from one type to another. This is useful when you need to work with values that are not of the expected type, or when you need to convert a value to a specific type for a specific purpose.
Example 1:
let name: string = 'John';
let age: number = 30;
// Casting a value from one type to another
let nameAsNumber: number = <number>name;
console.log(nameAsNumber); // Output: NaN
// Casting a value to a specific type
let ageAsString: string = age.toString();
console.log(ageAsString); // Output: "30"
Example 2:
let str: string = '123';
let num: number = <number>str;
console.log(num); // Output: 123
Example 3:
let arr: number[] = [1, 2, 3];
let str: string = <string>arr.join(',');
console.log(str); // Output: "1,2,3"
Example 4:
let obj: { name: string, age: number } = { name: 'John', age: 30 };
let name: string = <string>obj.name;
console.log(name); // Output: "John"
Example 5:
let value: any = 'hello';
let str: string = value as string;
console.log(str); // Output: "hello"
Example 6:
function isString<T>(value: T): value is string {
return typeof value === 'string';
}
let value: any = 'hello';
if (isString(value)) {
let str: string = value as string;
console.log(str); // Output: "hello"
}
TypeScript casting is a powerful tool that can help you improve the type safety and flexibility of your code. By explicitly converting values to specific types, you can ensure that your code is type-safe and maintainable. With its simple syntax and flexible options, TypeScript casting is a must-have tool for any developer working with TypeScript.