Using the Intl Object in TypeScript: An Overview with Examples
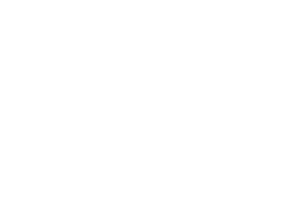
The Intl object in JavaScript and TypeScript provides a suite of tools for internationalization, allowing you to format numbers, dates, and strings according to various locales. It includes several constructors such as Intl.NumberFormat, Intl.DateTimeFormat, and Intl.Collator.
1. Number Formatting with Intl.NumberFormat
Intl.NumberFormat is used to format numbers, including currencies and percentages, in a locale-sensitive manner.
const number = 1234567.89;
// US English formatting
const usFormatter = new Intl.NumberFormat('en-US');
console.log(usFormatter.format(number)); // Output: 1,234,567.89
2. Date and Time Formatting with Intl.DateTimeFormat
Intl.DateTimeFormat formats dates and times in a locale-sensitive manner.
const date = new Date('2024-06-06T12:00:00Z');
const customDateFormatter = new Intl.DateTimeFormat('en-US', {
weekday: 'long',
year: 'numeric',
month: 'long',
day: 'numeric',
});
console.log(customDateFormatter.format(date));
3. List Formatting with Intl.ListFormat
Intl.ListFormat is used to format lists of items in a locale-sensitive manner.
const items = ['Apple', 'Orange', 'Banana'];
const enListFormatter = new Intl.ListFormat('en', { style: 'long', type: 'conjunction' });
console.log(enListFormatter.format(items)); // Output: Apple, Orange, and Banana
The Intl object in TypeScript provides powerful tools for internationalizing applications, making it easy to format numbers, dates, and strings according to different locales. By leveraging these tools, developers can ensure their applications are accessible and user-friendly to a global audience.