When to Use let vs const in TypeScript
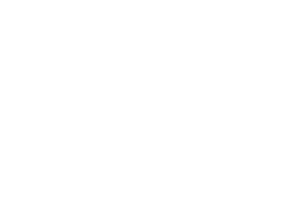
Understanding when to use let versus const in TypeScript is essential for writing clean, maintainable, and error-free code. Both let and const are used to declare variables, but they have distinct differences that influence how and when they should be used. This article will explore the best practices for using let and const, with insights from industry experts Dave Herman and Ryan Florence.
let
The let keyword is used to declare variables that can be reassigned. It is block-scoped, meaning the variable is only accessible within the block it was declared in.
let counter = 0;
if (true) {
let counter = 1;
console.log(counter); // Output: 1
}
console.log(counter); // Output: 0
const
The const keyword is used to declare variables that cannot be reassigned. Like let, const is also block-scoped. However, it does not mean the value it holds is immutable. Objects and arrays declared with const can still be modified.
const PI = 3.14;
PI = 3.14159; // Error: Assignment to constant variable.
const numbers = [1, 2, 3];
numbers.push(4); // This is allowed.
console.log(numbers); // Output: [1, 2, 3, 4]
Dave Herman, the author of "Effective JavaScript," advocates for using const by default and resorting to let only when reassignment is necessary. He says, "Using const is a powerful way to signal your intent and reduce the cognitive load on readers of your code."
In TypeScript, the choice between let and const boils down to intent and necessity. Use const by default to ensure variables are not reassigned, which leads to more predictable and maintainable code. Opt for let when you know that the variable's value will need to change. Following these best practices, inspired by industry experts like Dave Herman and Ryan Florence, will help you write cleaner, more robust TypeScript code.